What are you required to complete to create an application that is able to recognize living objects with React? (r) (r)
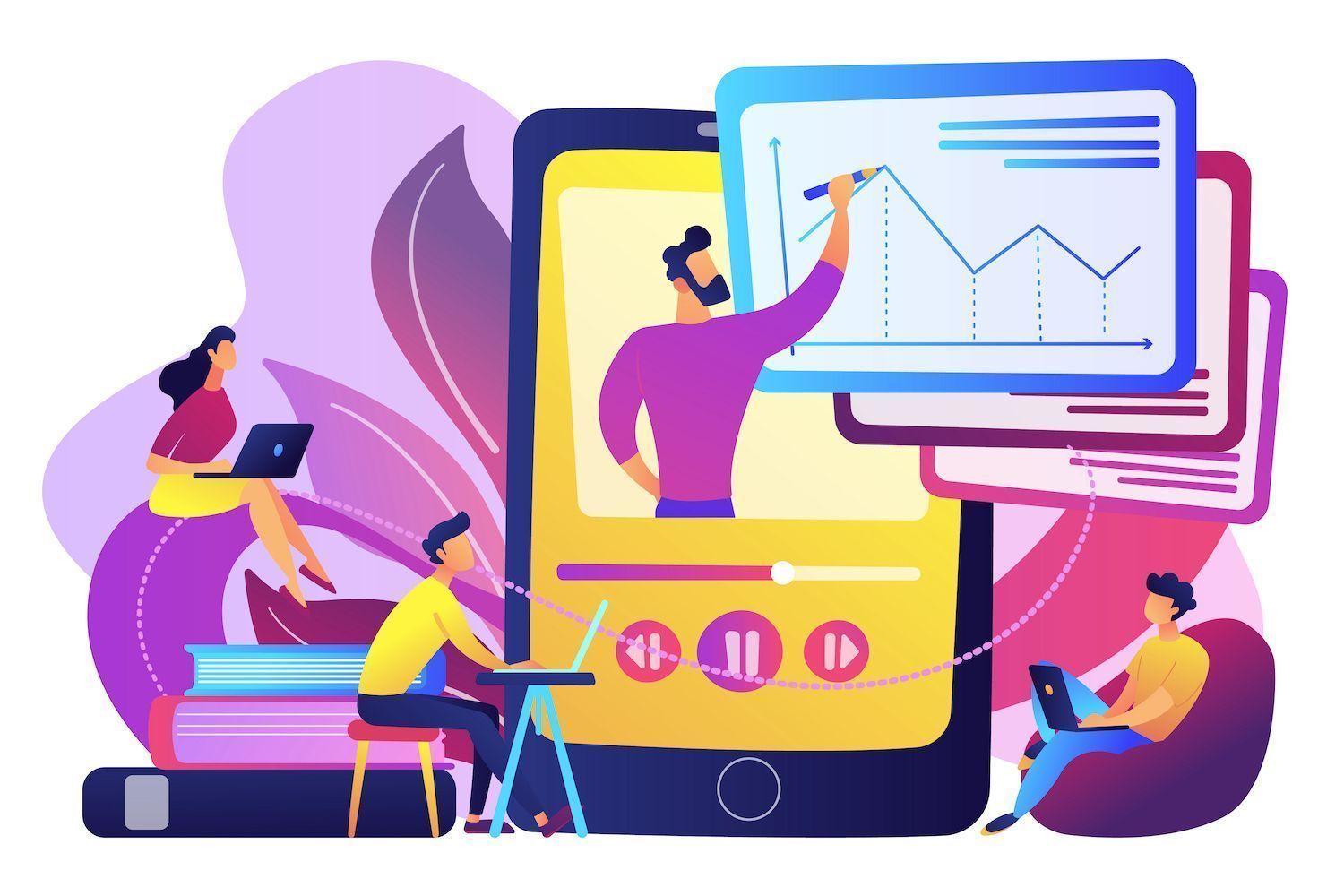
-sidebar-toc>
Cameras are getting more advanced and are becoming more sophisticated. the ability to detect objects in real time is becoming a more well-known option. From autonomous vehicles to advanced surveillance systems to AR applications, this technology is used to a wide range of uses.
Computer Vision is the nebulous phrase used to describe an approach that makes use of cameras and computers for various tasks. This is, as we've said previously, an extensive and intricate field. Many people do not believe that they are able to start participating in the immediate searching for items using your search engine.
The scene
The following is an extensive overview of the key techniques used in this article:
- TensorFlow.js: TensorFlow.js is a JavaScript library that brings the benefits of machine learning to your web browser. It lets you download existing models that have been trained to perform the task of object recognition, and then make them available to your web browser. It removes the need to perform complex server-side processing.
- Coco SSD It's an object recognition software that is trained. It's also known by the name of Coco SSD which is a light model that is capable to recognize the majority of the objects that are commonly utilized. While Coco SSD is a powerful tool, you need to recognize its reality that it was developed with a wide range of objects. If you're seeking an exact feature that you can detect then you can build an individual model using TensorFlow.js using this instructional.
Create a brand new React project
- Make a brand fresh React project. This is easy to accomplish by following these steps:
NPM create vite@latest -object detection React template
It will create the first React project that you can to build with with the aid of..
- After that, you'll be able to download TensorFlow and Coco SSD. Coco SSD libraries with these commands inside the project:
npm i @tensorflow-models/coco-ssd @tensorflow/tfjs
The time is now to build your app.
Configuring the application
Before writing the code that creates the logic necessary to recognize objects, have a look at the code that was written in this instruction. The user interface of this application could include:
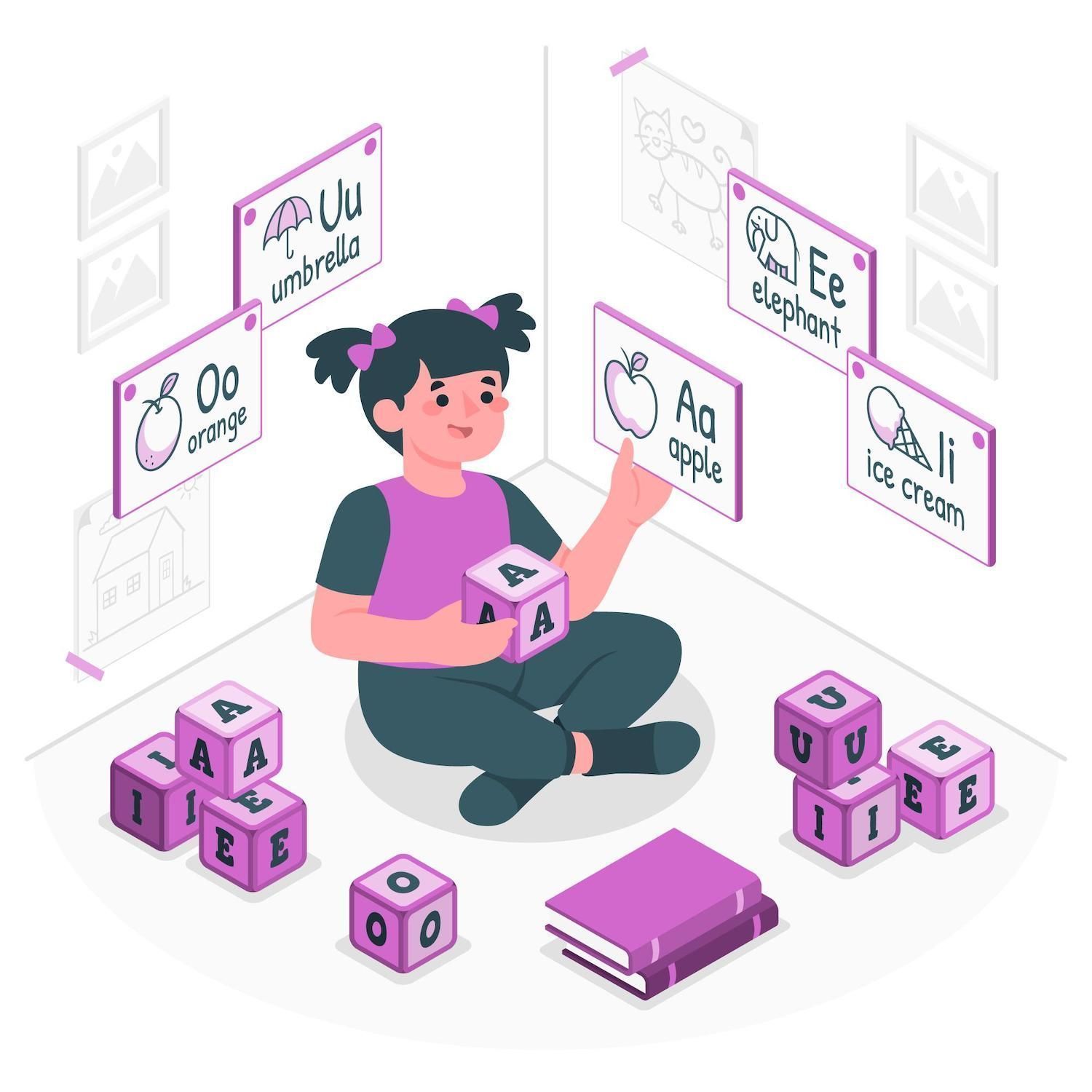
When users press the Start Webcam button following after clicking the Start Webcam button, they're asked to grant permission for the application to utilize webcam feeds. If permission is granted, the app begins to display the live stream from the webcam. It is also able to identify any things it observes in the stream. It then creates an equilateral triangle to show what it sees within the feed and it is able to label these items. It then is able to label the items.
First thing you need to do is to create a friendly user interface for your application. Then copy these actions to app.jsx. App.jsx file:
import ObjectDetection from './ObjectDetection'function App() return ( Image Object Detection ); Export default App
The code fragment acts as a page's header. The code also contains a custom component called "ObjectDetection"
. It takes the feed from a camera and identify objects at the spur of the moment.
To build this component make a new document with the name ObjectDetection.jsx in your homedirectory then paste the following code into it:
UseEffect, useState and'react'. Const objectDetection = () Const videoRef = useRef(null) Const [isWebcamStartedsetIsWebcamStarted], useState(false) Const setWebcam to be in sync () = /StopWebcam () > // TODO; return ( isWebcamStarted? "Stop" : "Start" Webcam isWebcamStarted ? : ); ; export default ObjectDetection;
You can implement the code in order to build startWebcam. "startWebcam"
function:
const startWebcam = async () => try setIsWebcamStarted(true) const stream = await navigator.mediaDevices.getUserMedia( video: true ); if (videoRef.current) videoRef.current.srcObject = stream; catch (error) setIsWebcamStarted(false) console.error('Error accessing webcam:', error); ;
The system will ask users to authorize access to their camera. When granted the system alters the video stream of the webcam. video
will display the live feed of the webcam to anyone that is connected to the website.
If the application is not in a position to connect to the feed of the camera (possibly because of the absence of a webcam in the device or a different reason why the user was not granted the access) it will show an error message to the console. The console could display an error message, which can explain the root of this issue to the user.
The next step is replacing stopWebcam by the stopWebcam
function with this code:
const stopWebcam = () => const video = videoRef.current; if (video) const stream = video.srcObject; const tracks = stream.getTracks(); tracks.forEach((track) => track.stop(); ); video.srcObject = null; setPredictions([]) setIsWebcamStarted(false) ;
The code scans for videos which are available through webcam objects. webcam
object. It then stops each one. After that, it will change to change the isWebcamStarted
status to the actual amount.
.
The same applies to this situation. it's possible to open the application and check whether it is able to connect and display the feed from the webcam.
This code needs to be placed into your index.css file to ensure that the app looks exactly as the one you had earlier seen.
#root font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif; line-height: 1.5; font-weight: 400; color-scheme: light dark; color: rgba(255, 255, 255, 0.87); background-color: #242424; min-width: 100vw; min-height: 100vh; font-synthesis: none; text-rendering: optimizeLegibility; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; a font-weight: 500; color: #646cff; text-decoration: inherit; a:hover color: #535bf2; body margin: 0; display: flex; place-items: center; min-width: 100vw; min-height: 100vh; h1 font-size: 3.2em; line-height: 1.1; button border-radius: 8px; border: 1px solid transparent; padding: 0.6em 1.2em; font-size: 1em; font-weight: 500; font-family: inherit; background-color: #1a1a1a; cursor: pointer; transition: border-color 0.25s; button:hover border-color: #646cff; button:focus, button:focus-visible outline: 4px auto -webkit-focus-ring-color; @media (prefers-color-scheme: light) :root color: #213547; background-color: #ffffff; a:hover color: #747bff; button background-color: #f9f9f9; .app width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: column; .object-detection width: 100%; display: flex; flex-direction: column; align-items: center; justify-content: center; .buttons width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: row; button margin: 2px; div margin: 4px;
The app.css file needs to be removed. App.css file to ensure that you don't harm the look of your parts. Now you are ready to implement the necessary technology of real-time object recognition into your application.
Implement real-time detection for objects
- Initial steps are to transfer the data into Tensorflow along with Coco SSD at the top of ObjectDetection.jsx :
import * as cocoSsd from '@tensorflow-models/coco-ssd'; import '@tensorflow/tfjs';
- Make a condition new in the
ObjectDetection
component, so as to preserve the prediction array generated by the Coco SSD model: Coco SSD model:
const [predictions setPredictions, useStatesetPredictions, useState ([]);
- After that, you are able to make an application which is loaded into the Coco SSD model. Coco SSD model. It loads into Coco SSD model, loads onto Coco SSD model, collects footage and predicts:
const predictObject = async () => const model = await cocoSsd.load(); model.detect(videoRef.current).then((predictions) => setPredictions(predictions); ) .catch(err => console.error(err) ); ;
The program uses footage from the video feed to generate forecasts about objects in the feed. The program provides users with an assortment of objects which are expected to be visible. Each one has a label which includes a percentage the level of confidence, and numbers that indicate the position of the object inside the video's frame.
It is vital to turn on this feature to process videos in the order that frames are added. The forecasts will be later used and kept in this forecasts
state. The forecasts will show boxes with labels for every identified object within the video stream present in the live view.
- Then you'll be able to position to utilize
this setInterval
function to trigger this function at a regular period. Also, you must be sure the function not active when the user has shut out of receiving updates through their webcam. To stop this from happening you must use theClearInterval
feature within JavaScript.Add your container for state and hooks for useEffect into theaffect
hooks in theelement, the detector of objects
element, to construct aprediction
function. This program runs continuously while the webcam is active, however it will then disappear from the webcam once it's turned off.
const [detectionInterval, setDetectionInterval] = useState() useEffect(() => if (isWebcamStarted) setDetectionInterval(setInterval(predictObject, 500)) else if (detectionInterval) clearInterval(detectionInterval) setDetectionInterval(null) , [isWebcamStarted])
It is developed to recognize objects in the image of the camera each 500 milliseconds. You can alter the number of milliseconds each second based on the speed you'd like the speed of object detection, but it is important to be aware of the possibility of applying the application frequently. The result could be the application taking up a significant chunk of memory inside the browser.
- When you've got the data to create your forecast you're now able to utilize the data to develop an estimate. If you've made some estimates of the forecast, this can be used to label containers. The forecast could be used for displaying the label on the container or to display the label on the live feed in the movie. For this to happen it is necessary to modify your
returning
declaration for yourlabels detection.
Enter the following details:
Return ( Is WebcamStarted ? "Stop" : "Start" Webcam isWebcamStarted ? : /* Add the tags below to show a label using the p element and a box using the div element */ predictions.length > 0 && ( predictions.map(prediction => return prediction.class + ' - with ' + Math.round(parseFloat(prediction.score) * 100) + '% confidence. ' > ) ) /* Add the tags below to show a list of predictions to user */ predictions.length > 0 && ( Predictions: predictions.map((prediction, index) => ( `$prediction.class ($(prediction.score * 100).toFixed(2)%)` )) ) );
The program displays the list of forecasts beneath the feed of the webcam. The program creates a empty space around the object that is forecasted with coordinates of Coco SSD as well as names on the bottom of every box.
- to style the labels and boxes correctly to style boxes and labels correctly, add this code within the index.css Index.css index.css file index.css file:
.feed position: relative; p position: absolute; padding: 5px; background-color: rgba(255, 111, 0, 0.85); color: #FFF; border: 1px dashed rgba(255, 255, 255, 0.7); z-index: 2; font-size: 12px; margin: 0; .marker background: rgba(0, 255, 0, 0.25); border: 1px dashed #fff; z-index: 1; position: absolute;
The application is fully completed. It is complete. The program has been capable of running the server which developed it to evaluate the functionality of the program. What happens after the program has been completed
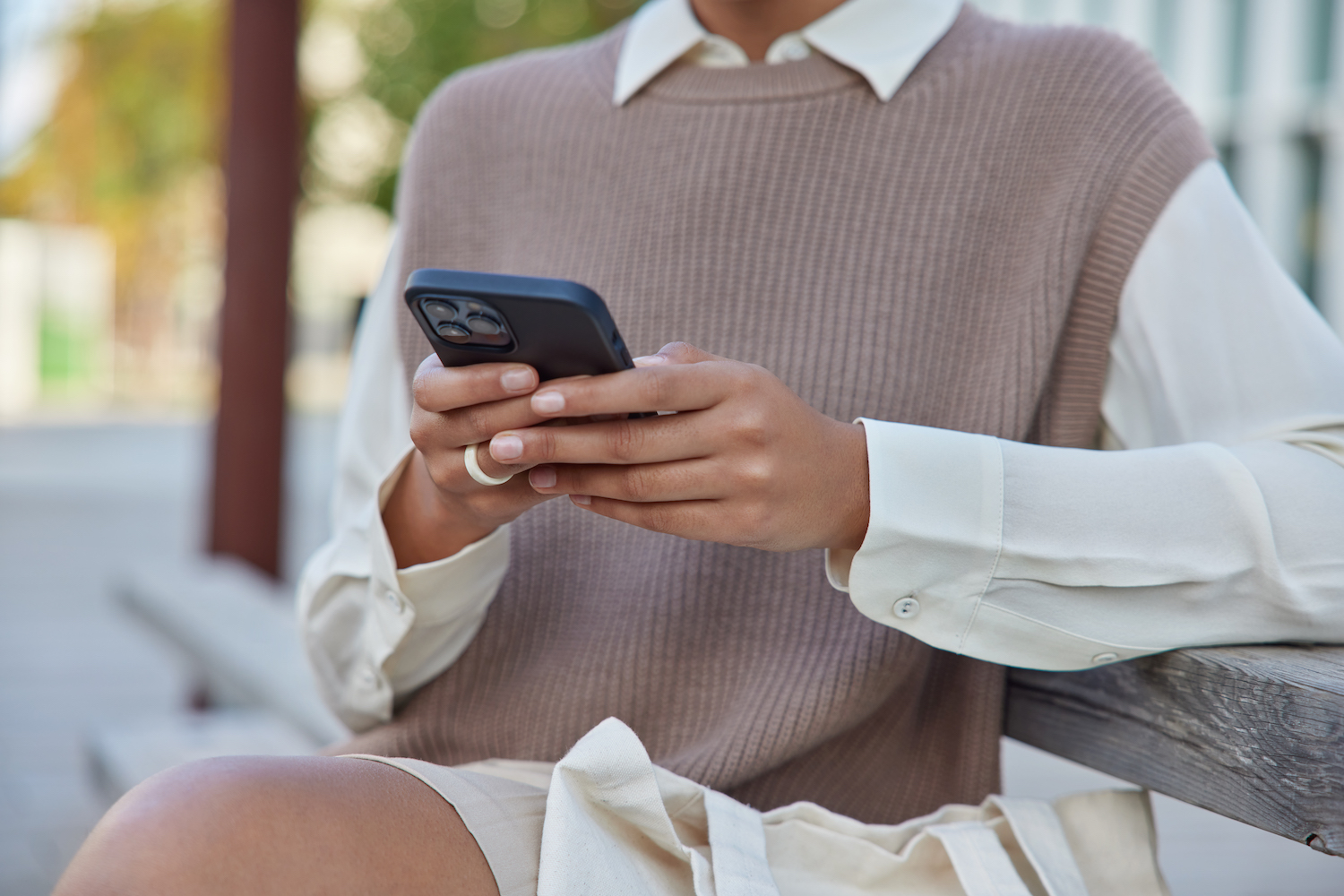
The complete code can be found on the repository at GitHub. GitHub repository.
Install the app
If your repository on Git is operational and functioning Follow these steps for installing the program :
- Sign up or create an account in order to access the dashboard at Your Dashboard. My dashboard.
- You must authorize your Git service provider.
- Select the static sites on the sidebar to left. Choose Add Site. Choose to Add the Website.
- Select the branch you wish to connect and the repository you wish to gain access to.
- Assign a unique name to your site.
- Set up the building's settings as per the following model:
- Command to build:
yarn build
orNPM build
- Node version:
20.2.0
- Publish directory:
dist
- Then, click Create site.
When the app is made, and the application has been rolled out then you are able to select "Visit the website" from the dashboard to start the app. The app can be tested with multiple cameras on various platforms to determine what it does.
Summary
It has had a huge success with creating the object recognition technology that is real-time in addition to live-time applications that is made with React, TensorFlow.js, and . This lets you explore the possibilities of computer vision, and develop interactive experiences through your browser.
Take note you are using the Coco SSD model we used is only an initial basis. If you'd like to keep looking into the various options, you should explore the possibility of being in a position modify the way of identifying objects with TensorFlow.js which allows you to alter the software to recognize those objects which best satisfy the particular requirements of the company you work for.
There's no limit on the possibilities you have! This app could be the foundation for creating advanced applications like Augmented Reality Experiences, as well as cutting-edge surveillance tools. If you're able to launch your app via the safe platform, you are capable of making your application accessible to anyone in the globe and witness the potentialities of computer vision rise to the spotlight.
What's your most challenging issue that you've faced which you believe that real-time detection of objects would be able to solve? Share your experience in the section of comments in the section below!
Kumar Harsh
Kumar is the writer of technical software. He is edits his home in India. He's an expert on JavaScript and DevOps. Find out more on his knowledge on his website.
The article originally appeared on on this site.
The article was published on this website.
The article was posted on this blog.
This post was posted on this site.
Article was first seen on here