What are the methods to debug Node.js Coding Using Multiple Tools
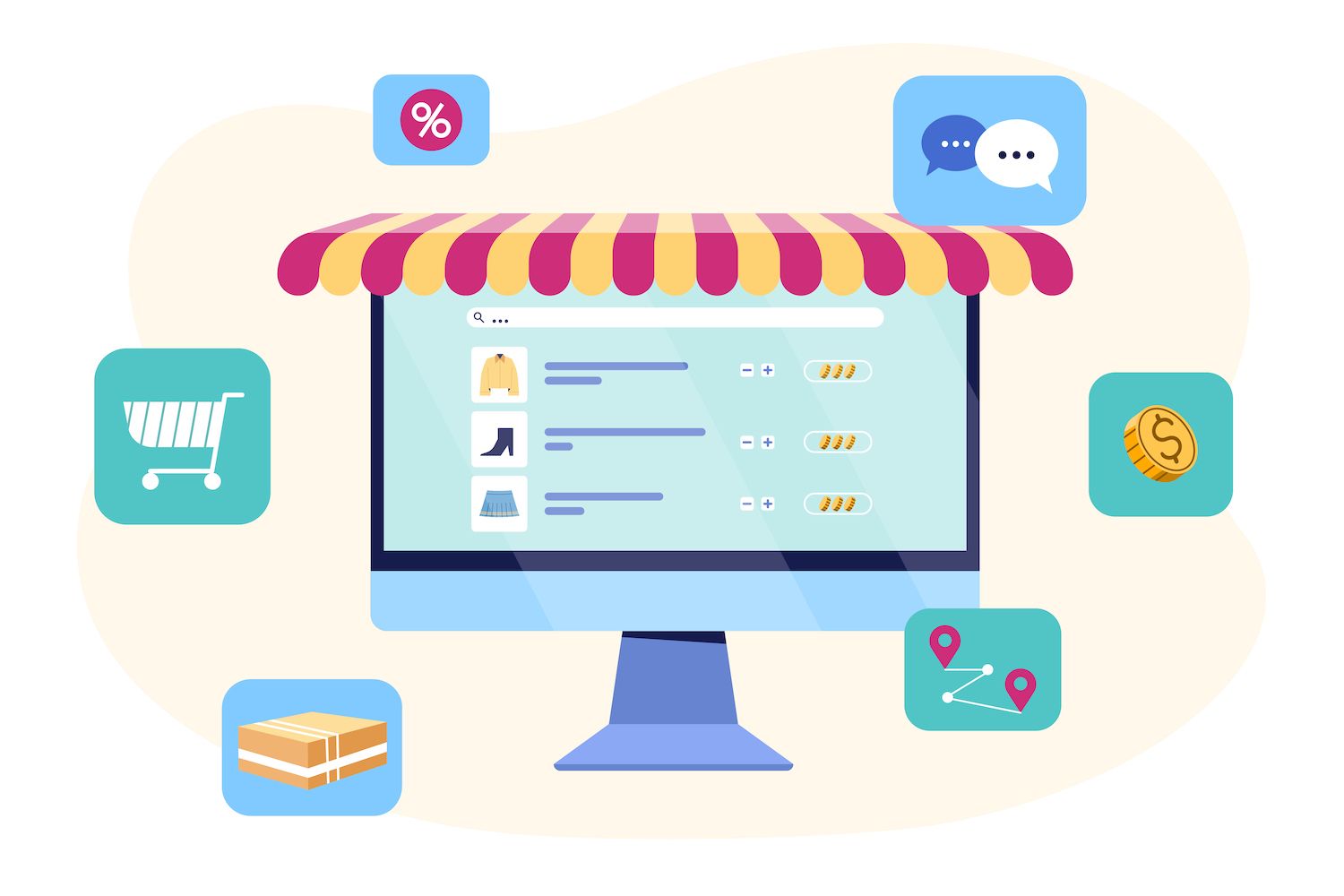
However, creating software may be a difficult task along with the Node.js code will fail at certain points. This guide will demonstrate the various tools used to detect errors in software and determine the root of the issue.
Let's begin.
Debugging Overview
"Debugging" refers to the term used to describe the various methods used to fix software problems. The process of solving problems is generally simple. However, the root cause could be more complicated and may require a lot of brain scratching.
In the following sections, we'll discuss three common types of errors you'll run into.
Syntax Errors
The code you create isn't in line with the conventions of the language, like when you take out the closing bracket, or mispelling a sentence such as console.lag(x)
.
A good code editor can help spot common problems by:
- False or legitimate statements are colored
- Type-checking variables
- Features for automatic completion and variables with names.
- The brackets should be highlighted to match.
- Auto-indenting code blocks
- The identification of codes that are not reachable
- The process of transforming complex tasks
A code linter like ESLint will also report syntax mistakes, incorrect indentation, and non-declared variables. ESLint can be described as the Node.js software you can download all over the world with:
npm i eslint -g
It is possible to check JavaScript documents from the command line using:
Eslint mycode.js
...but it's much easier to use the editor plugin , such as ESLint to Visual Studio Code and the linter-eslint plug-in for Atom which will instantly validate code as you type :
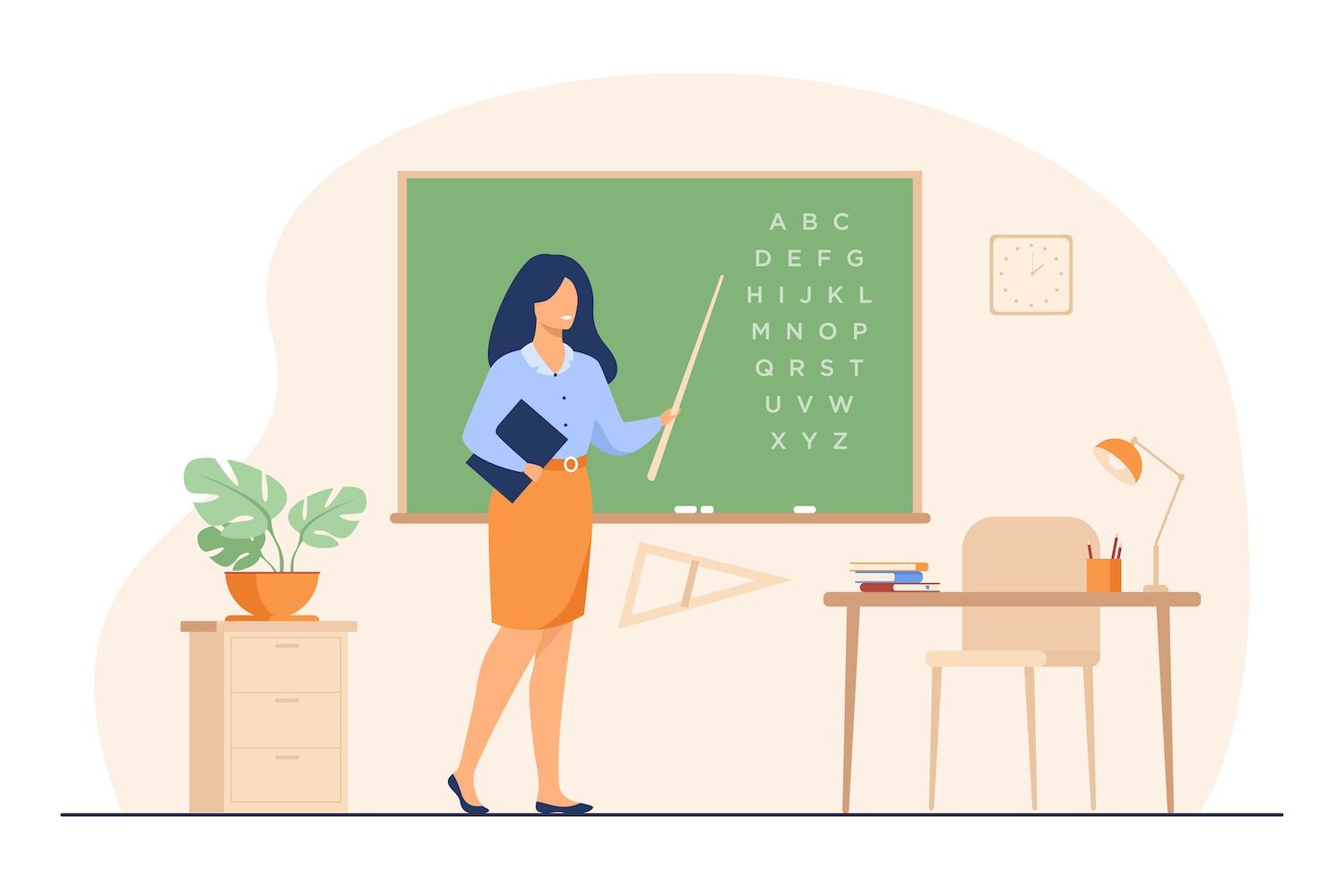
Errors in Logic
The program works, however it's not functioning as you'd like. For instance, the registration doesn't complete as they want it to be and the report shows wrong numbers, the data wasn't completely saved in the database, etc.
The cause of logic-related errors may be due to:
- The variable is not correctly used.
- Untrue conditions, e.g.
if (a higher than)
rather thanthe scenario of (a greater than 5)
- Calculations that don't include the priority of the operator e.g.
1+2*3
results in more than seven.
Runtime (or Execution) Errors
The error is only apparent once the program is run which can cause the program to crash. Errors during runtime may be caused by
- The variable that divides by is set to zero.
- The process involves accessing an array item that does not exist
- Write into the database which is read-only
Logical and time-related errors are harder to identify but the strategies listed below to develop can help:
- Utilize test-driven development TTD recommends that you develop tests before the moment that a function gets created, e.g. FunctionY will return X if Z is set for the parameters. Testing is conducted at the early stages of development and then later to verify that it is running as planned.
- Use an issue tracking softwareThere is nothing more frustrating than an email claiming "Your software is not able to function"! Issue tracking software allows you to keep track of certain issues, track reproduction steps, determine prioritization, assign developers and monitor the development of the solutions.
It's possible that you'll be encountering Node.js issues, however this section will give you ways to identify the issue.
Set Appropriate Node.js Environment Variables
Environment variables defined in the operating system running on the host could affect Node.js modules and programs. It is typically the NODE_ENV
which is normally switched to development when debugging or production mode when it is in the situation of being run in the server that is live. Create environment variables in macOS or Linux using:
NODE_ENV=development
or at or at or or at (classic) Windows command prompt:
Set NODE_ENV=development
Or Windows Powershell:
$env:NODE_ENV="development"
The popular Express.js framework making NODE_ENV a development blocks caches for template and displays errors in an ambiguous form this can be useful when trying to debug. Certain modules could provide similar functions and it's possible to include an option for NODE_ENV to your app, e.g.
// running in development mode? const devMode = (process.env.NODE_ENV !== 'production'); if (devMode) console.log('application is running in development mode');
Furthermore, you may take advantage of util.debuglog also. util.debuglog method to create error messages using an identified base e.g.
load debuglog from "util" MyappDebug const = debuglog('myapp'); myappDebug('log something');
The program only prints the log messages if NODE_DEBUG is set to myapp or a wildcard, such as my* or*.
Utilize Node.js Line Command Line Options
Node scripts are generally executed by node. This is then next followed by the title of the entry script.
Node app.js
Additionally, you can specify commands line options to regulate the different aspects of the timer. Useful flags for debugging include:
Check
Verify the syntax of the script prior to applying the scripttrace-warnings
produce a stack trace. This is the result of a situation where JavaScript Promises aren't able to solve the problem or deny--enable-source-maps
Source sources can be mapped using an application that converts for an example TypeScript--throw-deprecation
be alerted when warnings are issued when Node.js functions are used.--redirect-warnings=file
Input warnings to a file instead of warnings being sent to the file Not--trace-exit
produce a trace of the stack whenprocess.exit()
is executed.
Transmit messages to the Console
The ability to output a console signal is one of the easiest ways to verify an Node.js application.
console.log(`someVariable: $ someVariable `);
A few developers are aware there's a broad range of choices for consoles:
Console Method | Description |
---|---|
|
standard console message |
|
output object is the product from a very short JSON string |
|
properties of objects that print pretty |
|
output arrays and objects in tabular format |
|
an error message |
|
Counter's counter's increment, output and |
|
Create a counter with a particular value |
|
make an indentation on an email message |
|
Interrupting a whole group |
|
It starts an arranged timer |
|
Reports the time elapsed |
|
Stops a timer that is known as |
|
produce an output trace (a description of every function named) |
|
Clean the console |
console.log()
can also be used to accept an array of comma-separated numbers:
let x = 123; console.log('x:', x); // x: 123
...although ES6 destructuring offers similar outcomes, it requires lower effort
console.log( x ); // x: 123
Console.dir() can be described as a command which displays the property of objects. console.dir() command prints properties of object similar to util.inspect():
console.dir(myObject, depth: null, color: true );
Console Controversy
A few developers advise not use console.log() if you don't want to make use of console.log()
due to:
- There are many ways to modify things or you could ignore the need to get rid of it and
- There's no need to do it when you have better alternatives to debug.
Do not be deceived by someone who claims they don't utilize console.log()
! Logging can be an arduous and time-consuming process. But, everybody has to log in at least once throughout their lives. Make use of the technique or the tool you prefer. Repairing bugs is more important than the technique which you employ to determine the issue.
Make use of a third-party system for logging
Third-party log systems offer advanced capabilities like levels of messages, volume, sorting, file output and profiling, along with reports, and many more. Most popular choices are the loglevel system, pino cabin, morgan, Signale as well as the storyboard tracer, and winston.
Make use of the V8 Inspector
The V8 JavaScript engine includes the Debugging software which you can utilize to run a test of your program with Node.js. Start an application using node inspect, e.g.
node check app.js
The debugger stops the debugging process when it reaches the point where the line is finished. Debug> prompt
$ node inspect .\mycode.js One const number = 10. 3. (i = 0, (i
To get help, select Help to open the command lists. It is possible to navigate within the program using:
- or C: continue execution ou C for C: continue execution
- next or the next to perform the next command
- step or step step or step functionality described as
- removed or out or Move away from the operation, and come back to the call statement
- pause: pause running code
- watch('myvar') Check the variable
- setBreakPoint() or sb(): set a breakpoint
- restart Start the script
- .exit or Ctrl Cmd exit the debugger
Debugging can be time-consuming and unwieldy. Make use of it only when you have another option, such as, when you're working on software that's running on an external server, and you're unable to connect elsewhere or install additional software.
Utilize the Chrome Browser for debugging Node.js Code
Do you want to know the methods we used to grow our volume by more than 1000 percent?
Join our 20,000+ subscribers members who receive our weekly newsletter which contains insider WordPress advice!
If you wish to investigate a typical web-based application, you must start it using inspect to enable the V8 Debugger's Web Socket server:
Node Inspect index.js
Note:
- index.js might represent the entry script for the application.
- Make sure you select
the option to inspect
using double dashes in order to ensure that you do not launch the client for debugging by making use of text. - You could make use of nodemon instead of node if you'd like to restart your application when the file has been modified.
The debugger will only allow connections from the local device. If you are running your program using a different device, virtual machine, and an Docker container, you can use:
node --inspect=0.0.0.0:9229 index.js
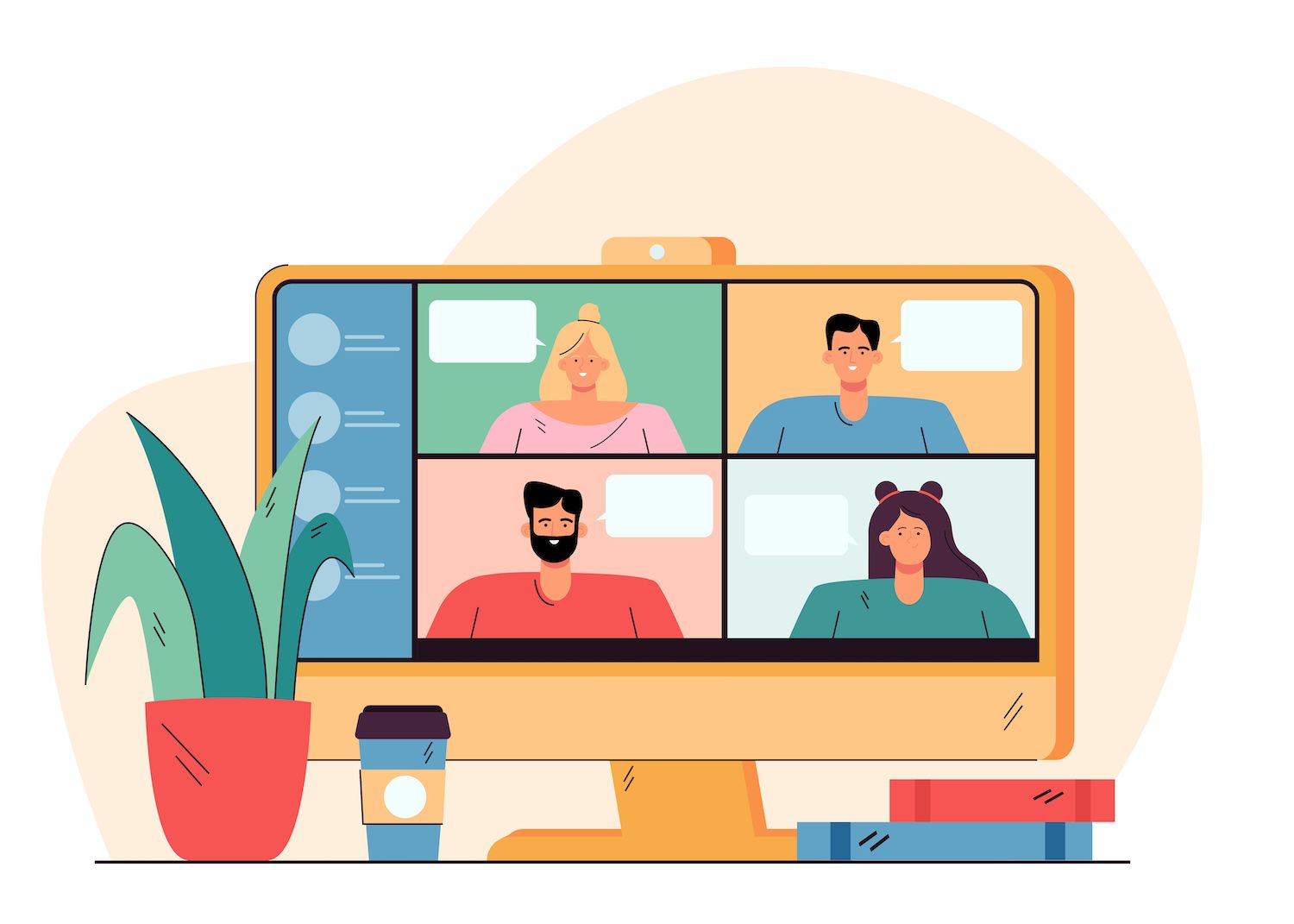
There is also the option to use the option --inspect-brk
instead of inspect
to halt running (set an breakpoint) within the first line. This lets you go through the code from beginning.
Start a new browser with Chrome and type Google://inspect
in the address bar. It will show the devices that are networked and local:
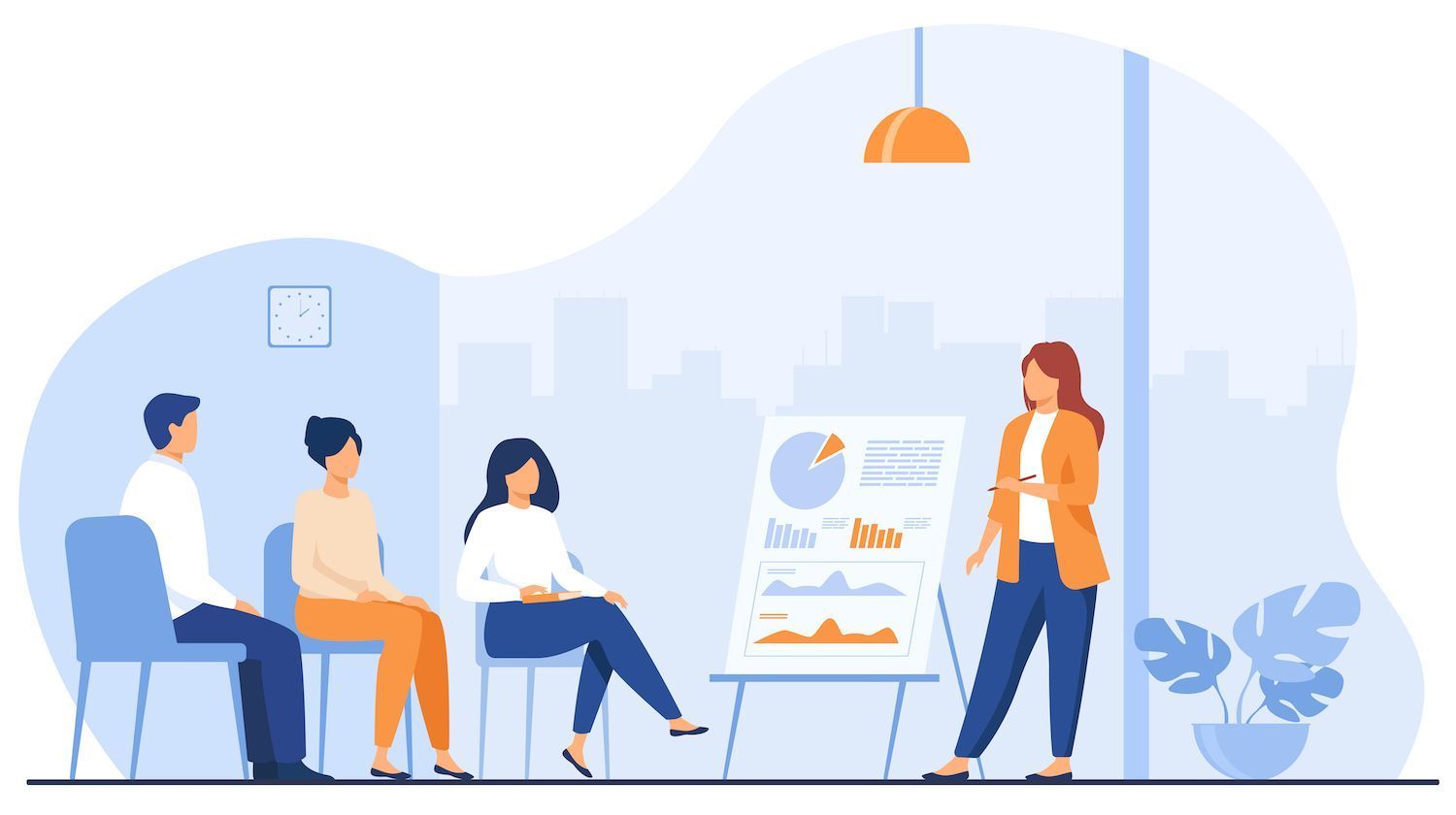
If your Node.js application isn't displaying as Remote Source, Remote Source or isn't able to find it:
- Choose Open the DevTools dedicated to Node and choose the address and port or
- Find the location of the network you'd like to discover. Click to configure to add the IP address as well as the port number for the device that it's running.
Use the destination's inspect link to start DevTools' DevTools Debugger Client. It should be well-known to any person who's used the DevTools tool to investigate client-side issues:
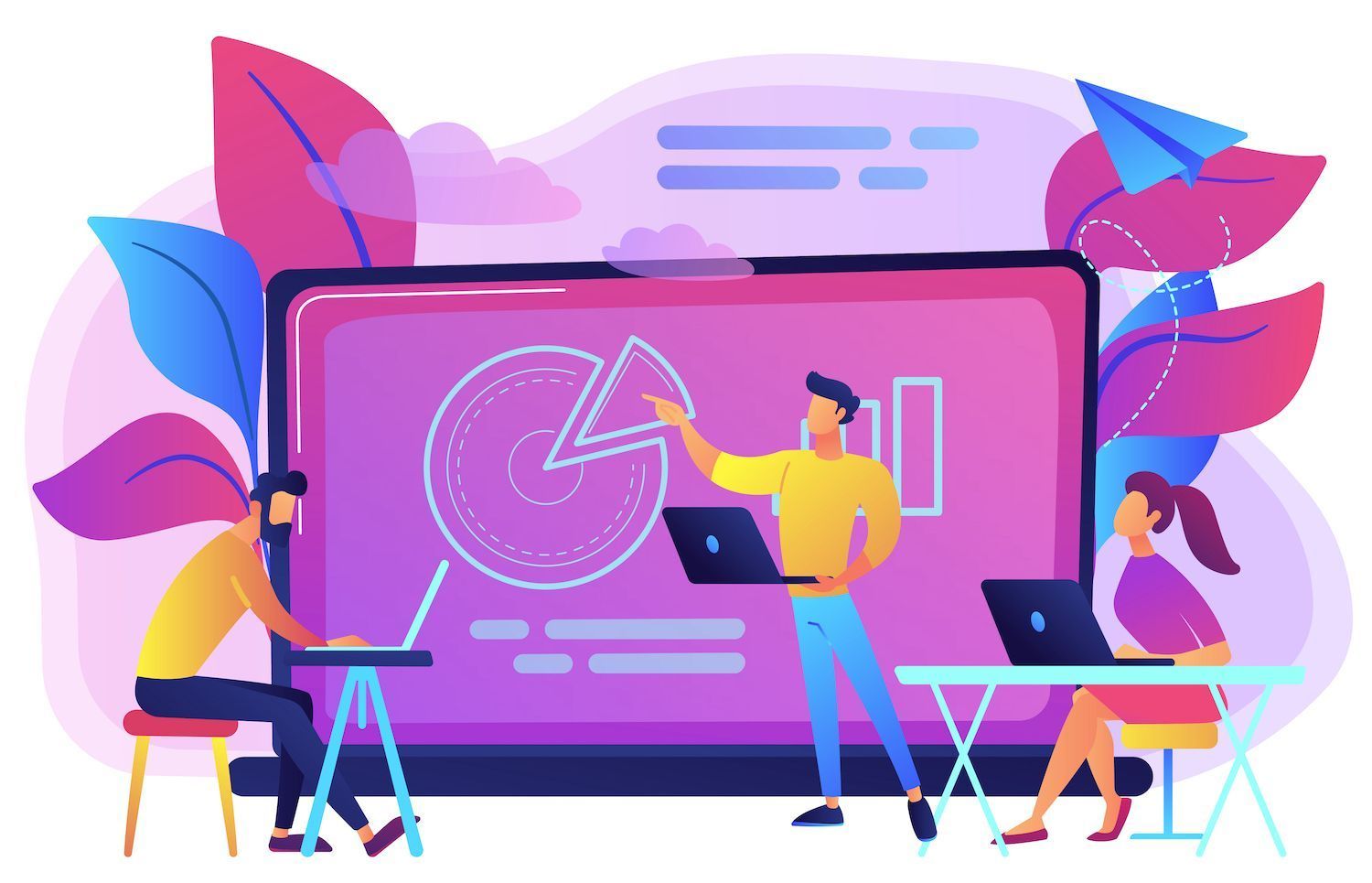
Change on your Sources panel. It is possible to start any file by pressing Cmd Ctrl + P, and after that entering the name of the file (such for example, index.js).
It's also simpler to connect your project's directory to the workspace. This lets you load files, modify and save your files right via DevTools (whether you consider that the right idea is a different matter!)
- Click + Add folder in the Workspace
- Choose the best address to host the project. Node.js project.
- Click Acceptto permit file modification to be completed
It is now possible to download files from the left directory tree:
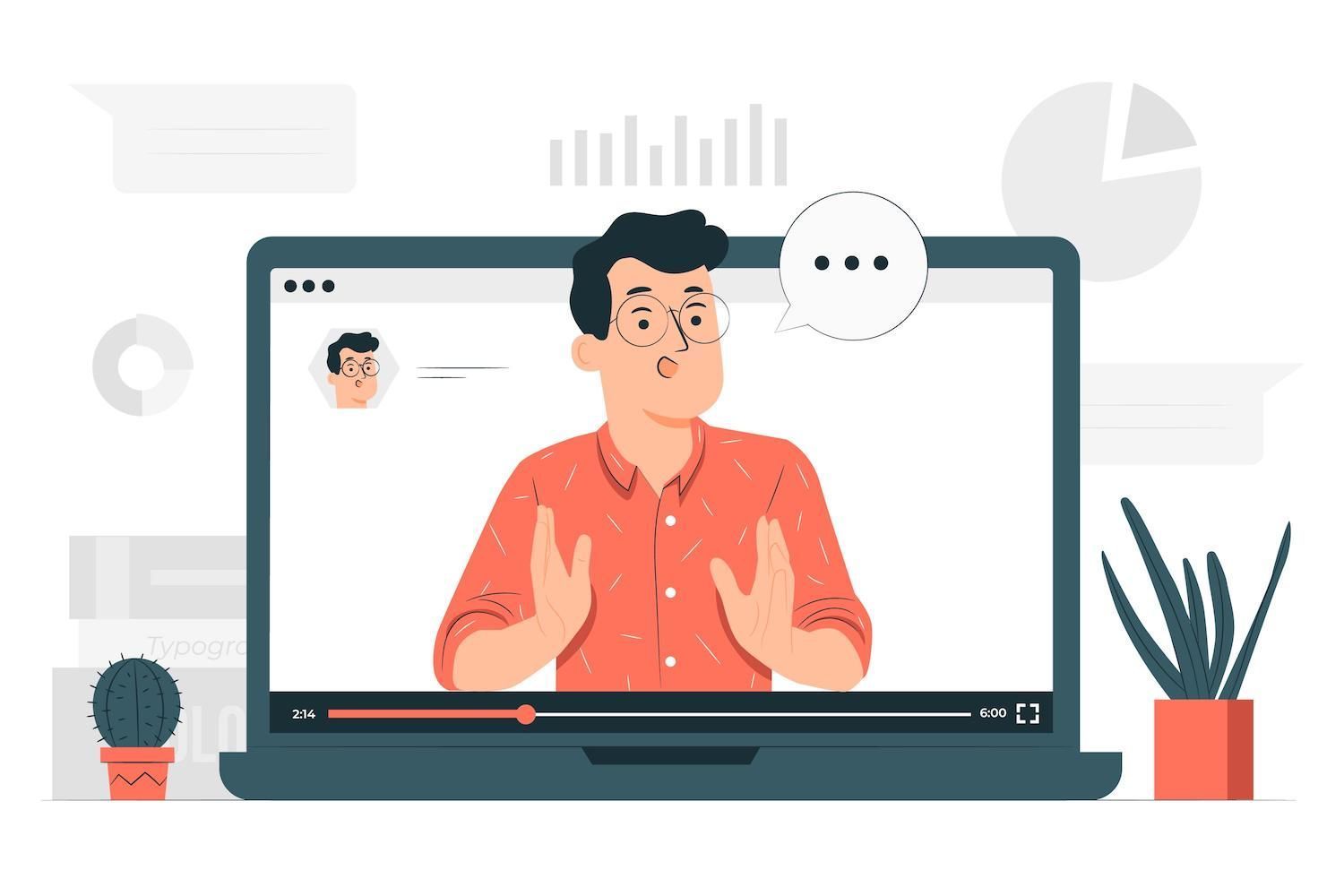
Simply click any line to create a breakpoint as indicated by the blue marker.
Debugging depends on breaks. Breakpoints are the point at which the debugger should stop program execution. They also indicate what is happening to the programmer (variables as well as call stacks, and the like.)
You are able to specify how many breakpoints you wish to include in your user's user interface. Another possibility is to include a debugger; statement into the code, which will stop once the debugger has been attached.
Use your browser's web interface to get to the line that a breakpoint was established. In the example here, http://localhost:3000/ is opened in any browser, and DevTools will halt execution on line 44:
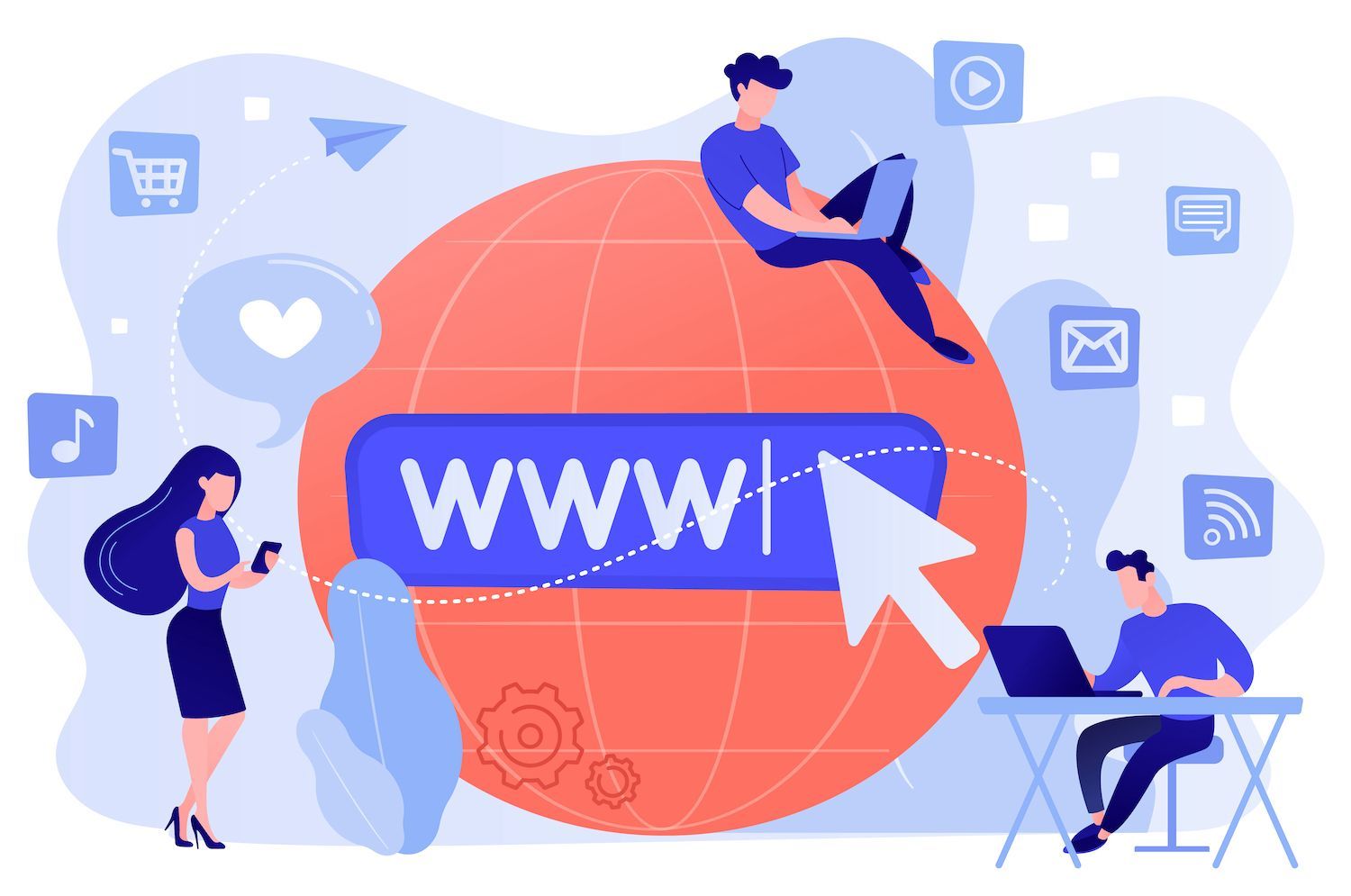
The left-hand side of the panel is a representation of:
- Action icons placed on the row (see the next section).
- Watch pane The Watch pane allows you to monitor variables using the + icon, then typing their names.
- The Breakpoints pane gives an overview of all breakpoints that are available, and permits the breakpoints to be disabled or turned on.
- Scope Pane The Scope pane provides the current state of every module, globally and locally. This is the pane is the one you'll see most frequently.
- An Call Stack pan is a list of the order of calls to be made at this point.
The row of icons for action is shown above The icons representing action can be paused when breakpoints occur.:
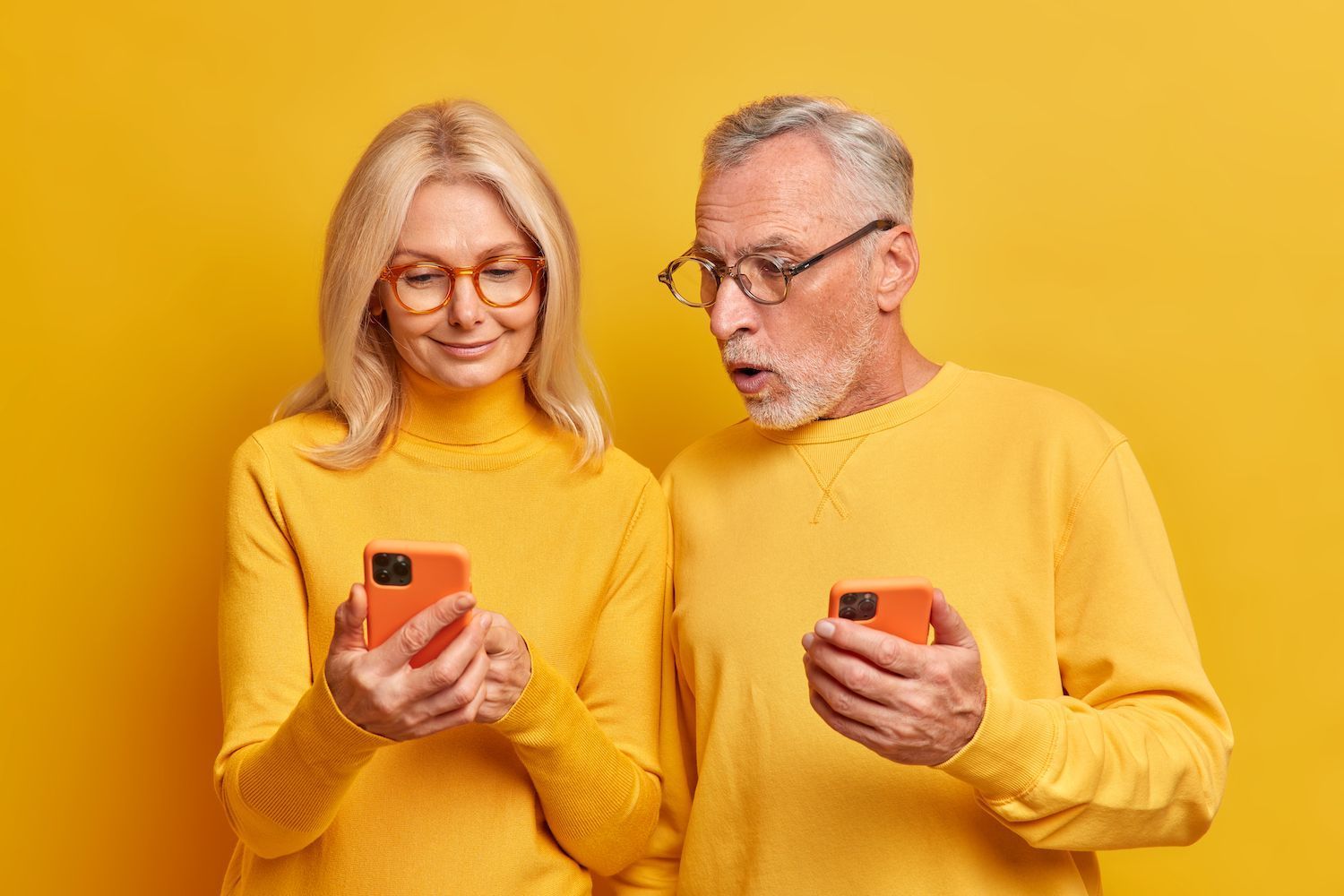
Starting from left and moving to the right, starting at the left
- Resume execution Keep working until the breakpoint is reached.
- step-over The following step is to execute the command, and remain inside the section of code in use at the moment. Don't dive into commands that it triggers.
- Step in Step in HTML0 Run this command, before moving on to the next task according to the need
- eliminate : Continue processing to the conclusion of the process before returning to the calling command
- step is similar as the step however, it does not have the ability to jump into the functions that are not async.
- remove all breakpoints
- Stops processing in the situation of an error. processing is stopped if the error is discovered.
Conditional Breakpoints
In some cases, it's necessary to have some more influence over breakpoints. Take a look at an example of a loop that's run 1000 times, and you're just interested in what happened in the initial one.
for (let i = 0; i
If you don't want to press to resume execution 99 times, by clicking on the line, you can select the option to create an a conditional breakpoint that will identify a specific situation, for example that the number i = 99
:
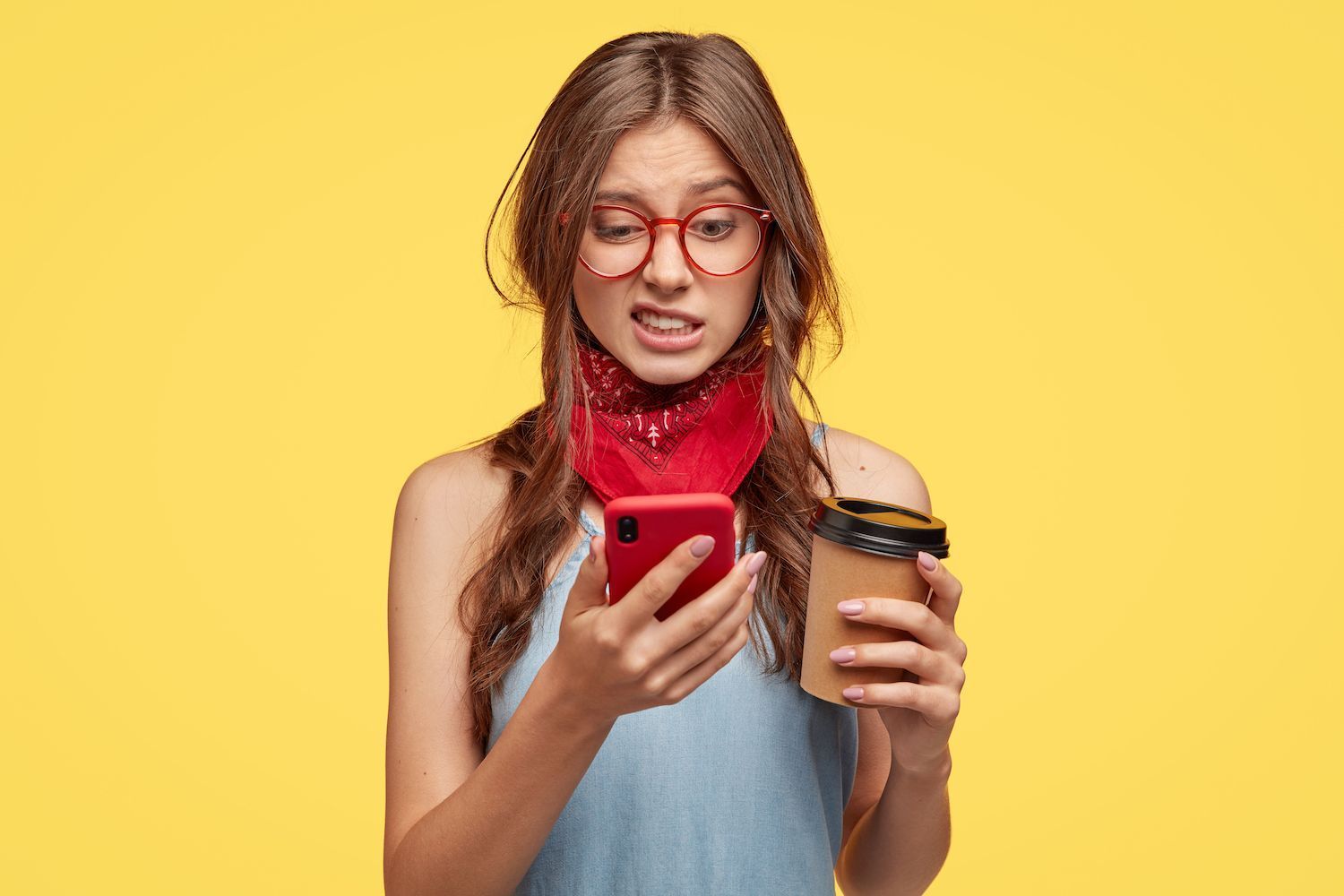
Chrome displays conditional breakpoints as blue and not yellow. That means the breakpoint will only be active after the completion in the loop.
Log Points
Log points effectively enable the creation of console.log() without writing code! Expressions are generated at any time the code runs every line. It will however not cease the process, just like an endpoint.
To make log points you need to right-click on any line, then select the option for creating an account, and then enter an expression. e.g. "loop counter"loop counter" (i)
:
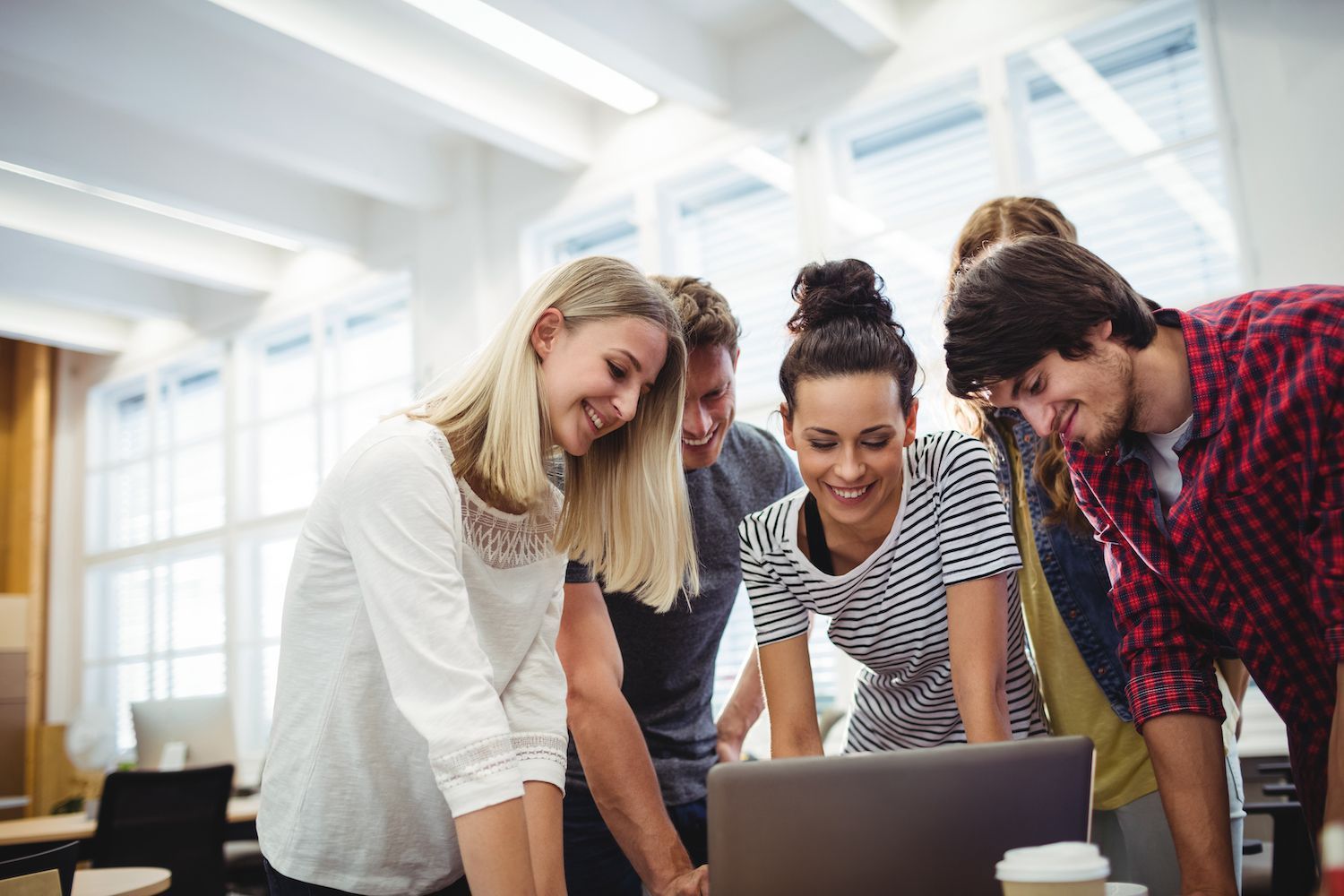
The DevTools console can output loop counters i.e. for loop counter i.e. 99 in the above example.
Use VS Code to Debug Node.js applications
VS Code can work with Node.js and comes with the Debugging tool. Many applications can be tested without the need for setup, and the editor will initiate the debugging servers and the client.
Then, open the beginning file (such such as index.js) after which, within the Run and Debuggle tab, hit the run and debug button and select to start to the Node.js environment. Select any line that you want to modify and then to create a breakpoint, which is highlighted with the red circle. After that, you can launch the application in the browser in the same way as before. However, VS Code ceases execution after it reaches the breakpoint.
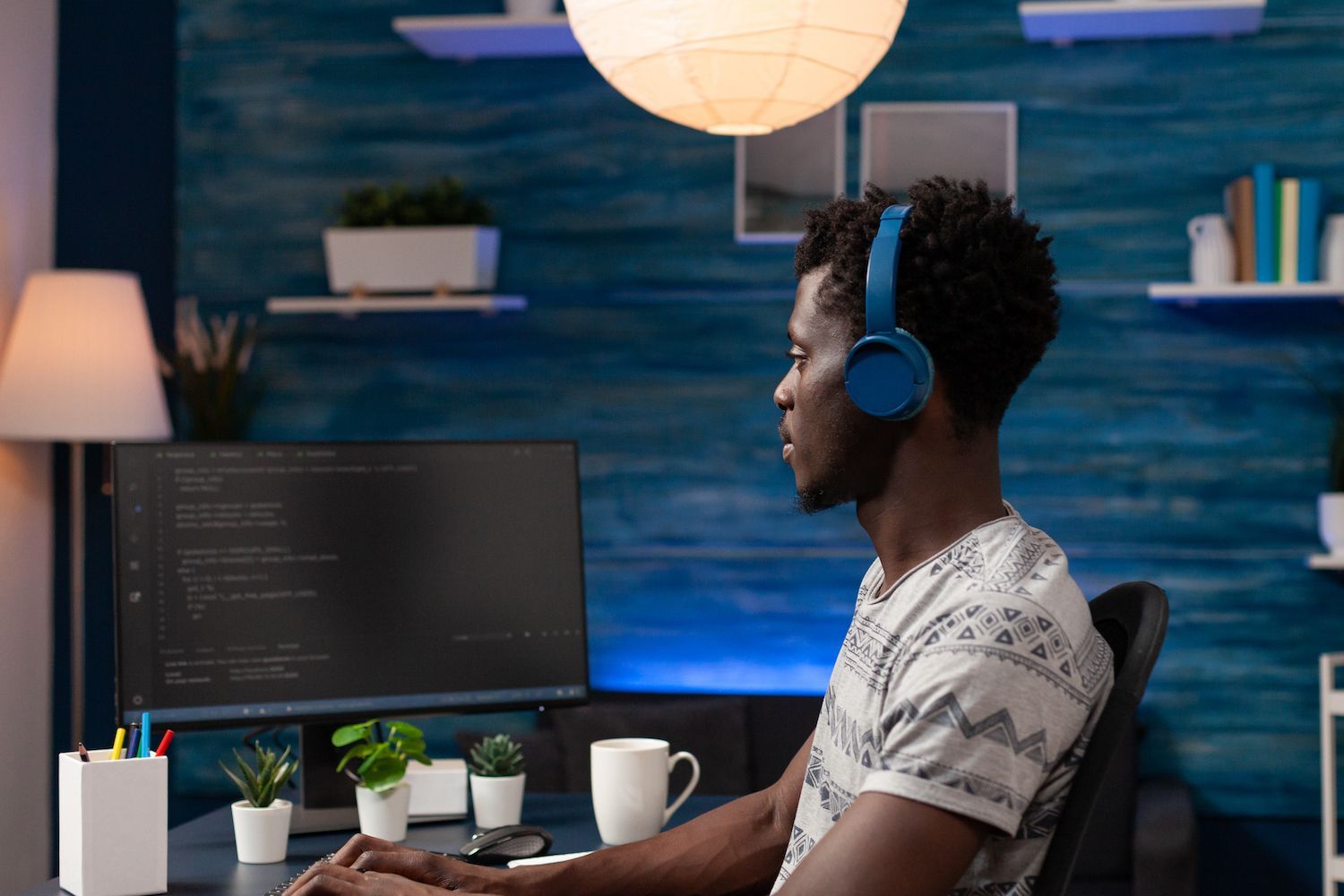
The toolbar with action icons can be utilized for:
- restart execution to continue processing till the following breakpoint
- Step Over step by step Follow these steps, however stay within the functions that you're using.Do not call the function that it invokes.
- Step to Step in: Type the command that follows, afterwards, you can jump into the program it calls
- Then step out and process until the end of the function and then return to the command line
- Startthe application, and the debugger
- Closethe application along with the debugger
Similar to Chrome DevTools, it is you can right-click on any line to include breakpoints that have conditional breaks in addition to the log point.
For more information, refer to Debugging in Visual Studio Code.
Advanced Debugging within VS Code Configuration
Furthermore, VS Code configuration may be necessary if you are planning to debug code on another gadget, such as a virtual machine or to utilize alternative launch options such as nodemon.
VS Code stores debugging configurations inside an launch.json file. It is stored within the .vscode
directory in the project. Begin by selecting the run as well as debugging tab. In order to create the launch.json file, opt to make use of the Node.js environment to make this file. An example configuration for this is available:
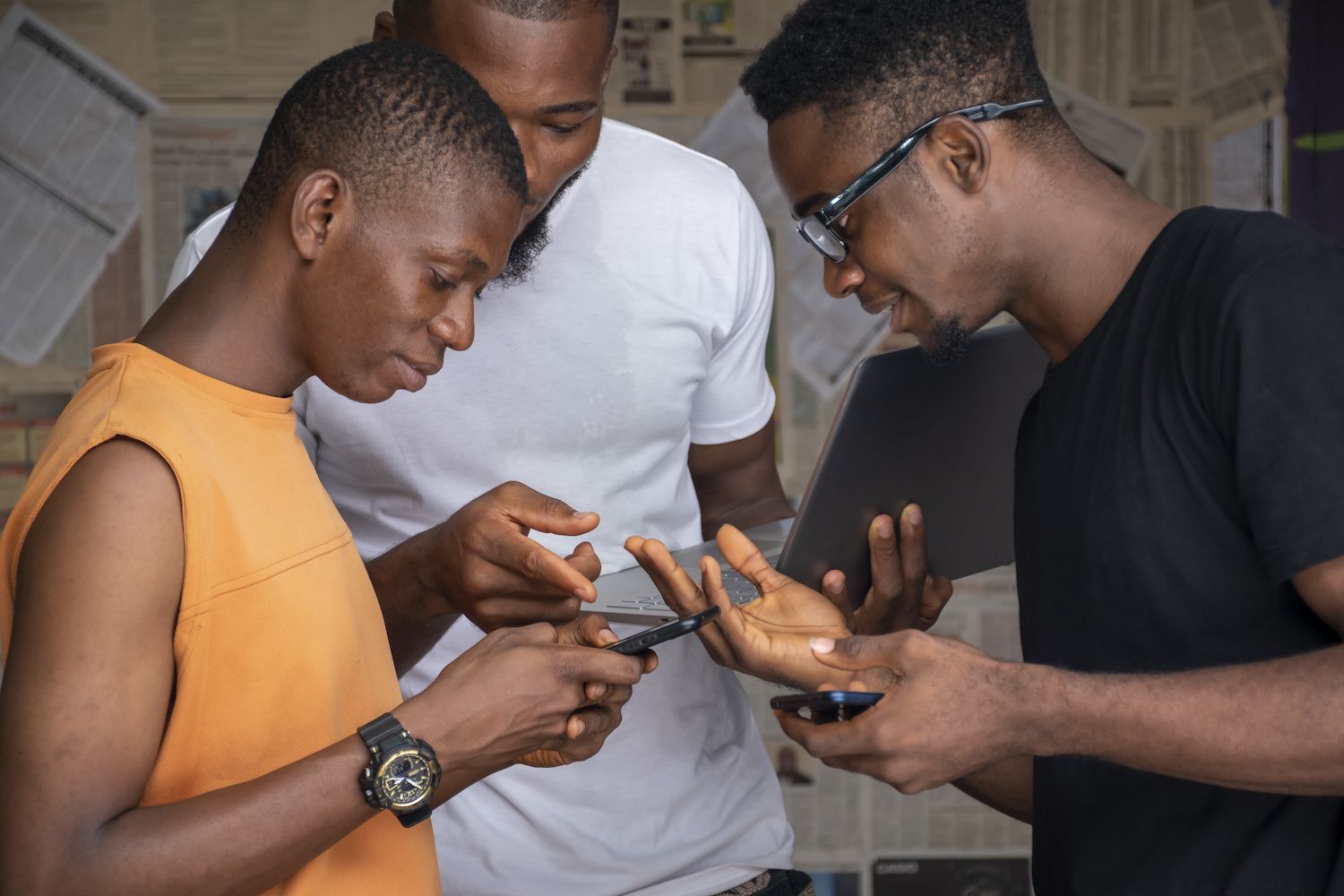
The different configuration options are accessible within the "configurations"
array. Select on the"Add Configuration... and select the right option.
An individual Node.js configuration can either:
- The process could be started by itself or
- Attach to the testing Web Socket server, perhaps that is running from a remote device, as well as a Docker container.
For example, to define an Nodemon configuration, you must choose Node.js Setting: Nodemon and then edit to alter"program" entry script "program" entry script according to what is required
// custom configuration "version": "0.2.0", "configurations": [ "console": "integratedTerminal", "internalConsoleOptions": "neverOpen", "name": "nodemon", "program": "$workspaceFolder/index.js", "request": "launch", "restart": true, "runtimeExecutable": "nodemon", "skipFiles": [ "/**" ], "type": "pwa-node" ]
The launch.json
file and the configuration for nodemon(the names of the settings "name") can be seen within the drop-down menu located to the right of the Run and Debug pane. Choose the green Run icon to turn on the configuration. Run the application by using the nodemon.
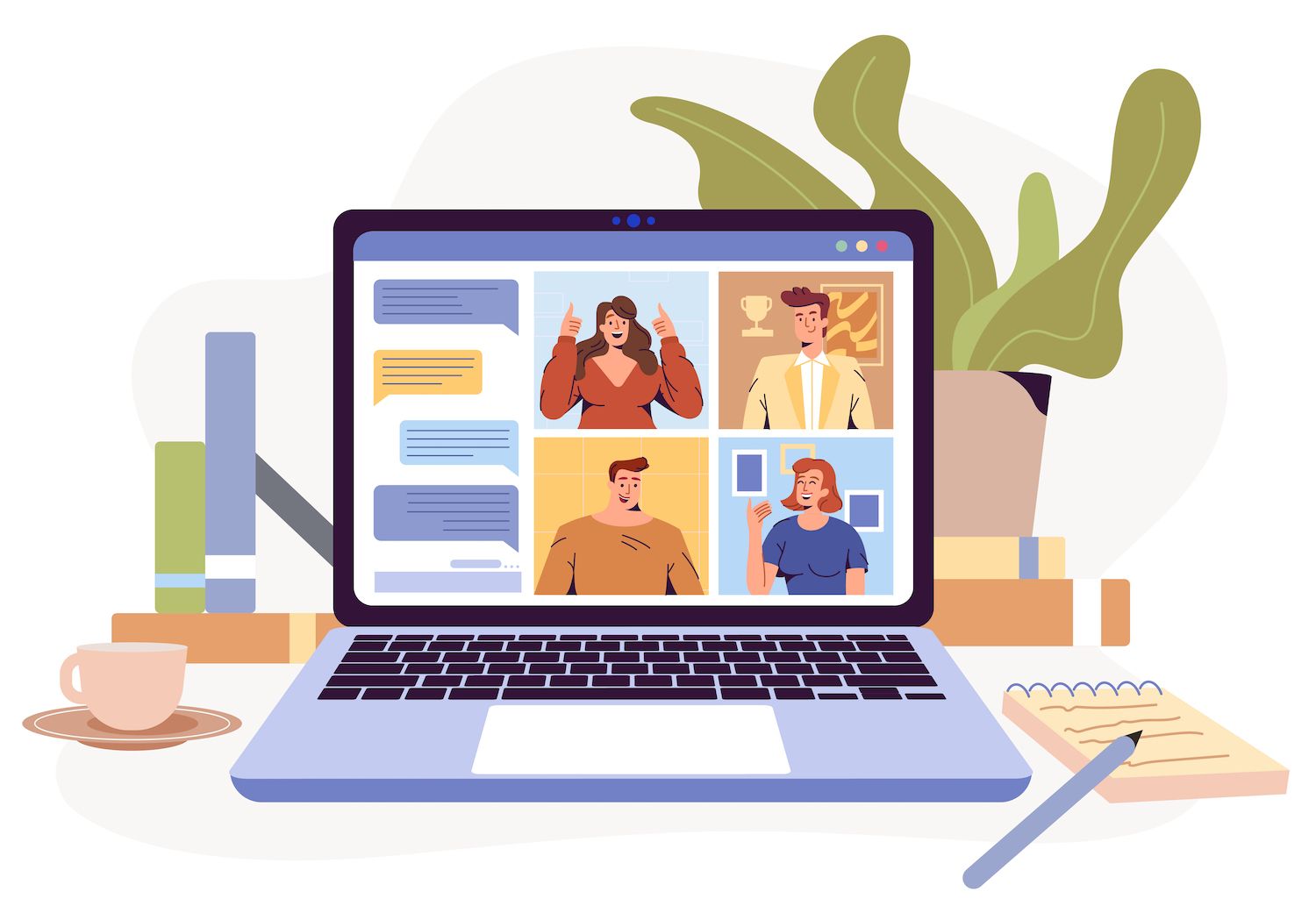
As before, you can create breakpoints, conditional breakspoints and logpoints. The main difference is that nodemon will start your server whenever the software's configuration is changed.
If you require more information or specifics, go to the settings for VS Code Launch.
The below VS Code extensions can also aid you in debugging code that is stored on isolated or remote servers:
- Remote Containers are connected to the apps running within Docker containers.
- Remote SSH Connect to programs that run on remote servers
- Remote WSL Connect to applications that run on Windows Subsystem for Linux (WSL). Windows Subsystem for Linux (WSL).
Others Node.js Debugging Options
The Node.js Debugging Manual provides advice for editors for text and IDEs, including Visual Studio, JetBrains WebStorm, Gitpod, and Eclipse. Atom comes with the Node-Debug plugin which integrates with the integrated Chrome DevTools debugger into the editor.
When your application has gone live You may want to think about using commercial debugging services such as LogRocket and Sentry.io, which allows you to record and replay error messages delivered to real-world customers.
Summary
Make use of any device you're capable of using to identify the issue. You can use console.log() to console.log() to accelerate bug finding, Chrome DebTools and VS Code may be preferable when dealing with more difficult difficulties. These tools can help you build more secure software. It allow you to spend less time attempting to solve issues.
What Node.js practices is your most trusted method? Do you have any suggestions? Please post them in the comments section below!
It is easier to save time, money and boost site performance:
- Support is immediately available from WordPress experts at every hour of the day.
- Cloudflare Enterprise integration.
- Global reach with 29 data centers spread across the globe.
- Optimization via the integrated Application to measure the effectiveness.
This post was posted on this website.
Sign up to our monthly newsletter
Subscribe to the latest blog posts right to your inbox. Your email address Subscribe
You must enter an email address with a valid validity
Oops! The email was not sent correctly. the email, please try later.
Article was first seen on here