Laravel API Create and test an API for Laravel -(r) (r)
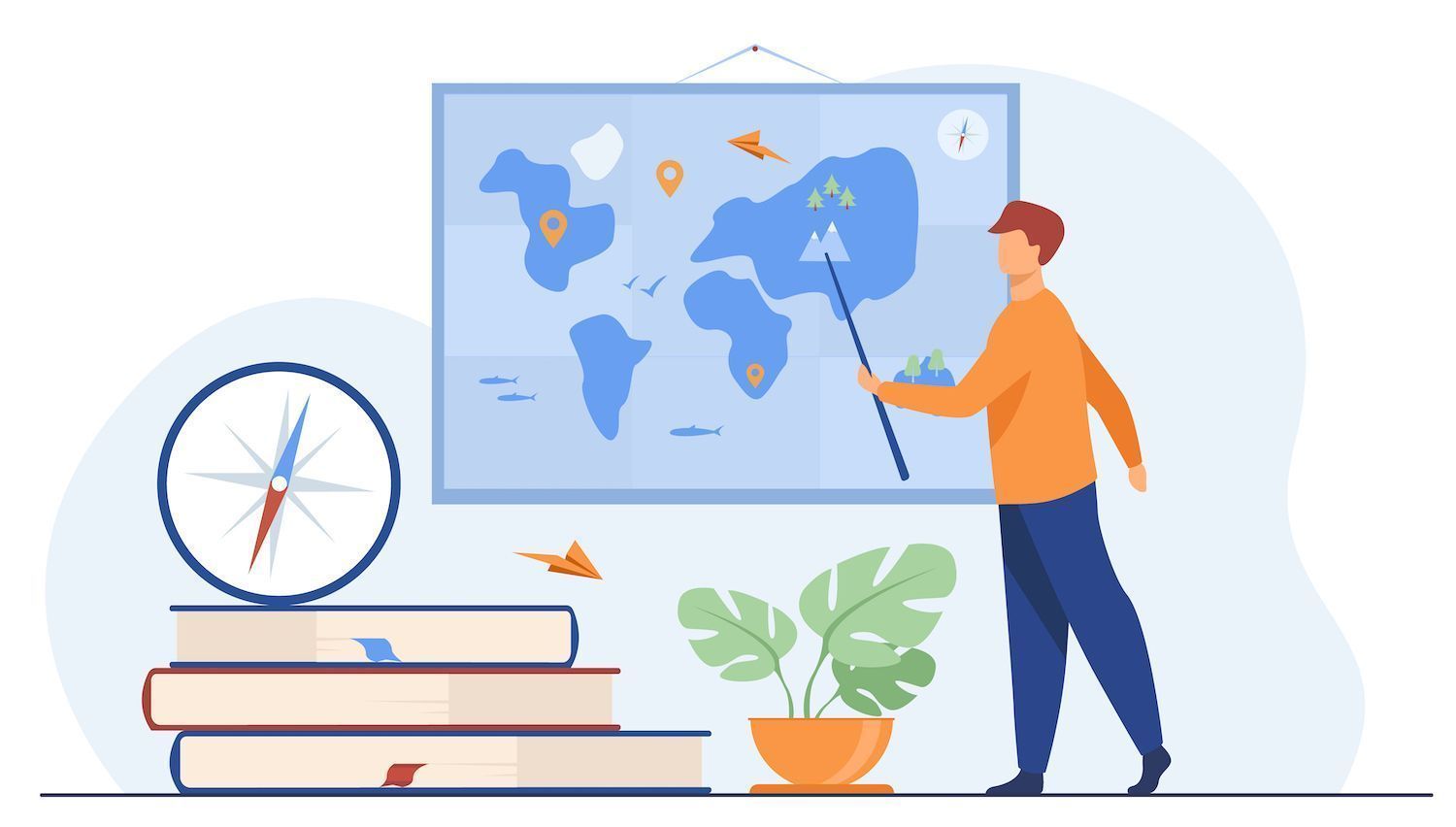
Send the information to
Laravel Eloquent is a simple approach to connecting your database. It's an object relational mapping (ORM) that reduces the complex database process by creating a framework that permits you to interact with tables.
Also, Laravel Eloquent has excellent tools to create and test APIs. These tools can assist users in developing APIs. In this guide, you'll learn how simple to create APIs and then test them in Laravel.
The circumstances
To get you started, the process, here's the information you'll need
- Version 8 of HTML0 is currently being made available for download with Laravel as well as version 9.
- Composer
- Postman
- XAMPP
API Basics
Make a modern Laravel project with an experienced composer
:
composer create-project laravel/laravel laravel-api-create-test
To begin the server begin the server, you must execute this command. The server is started at port 800:
cd laravel-api-create-test php artisan serve
It is highly recommended that you review the following screen:
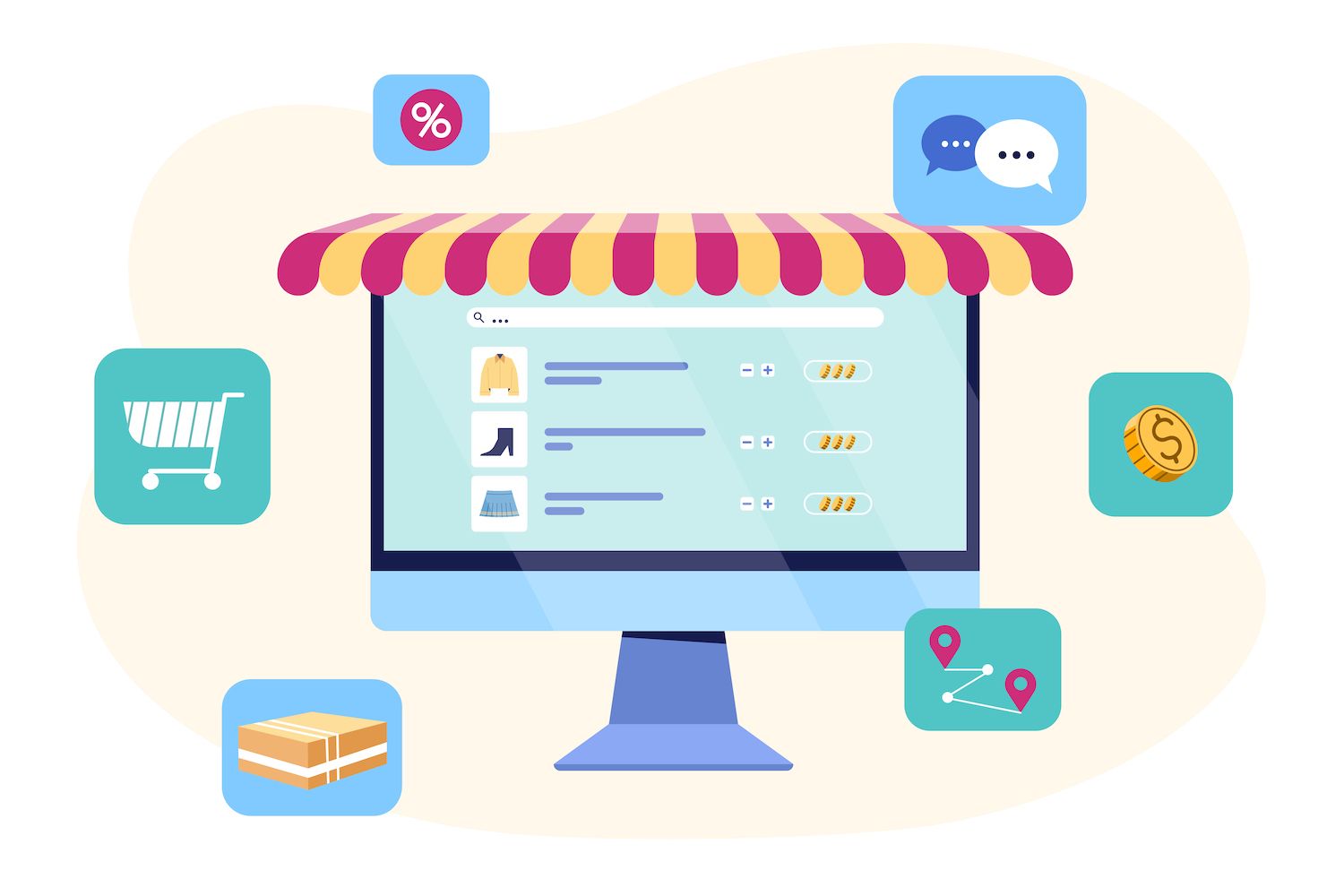
Models that are made with"-m" as their "-m
flag to allow the migration to be made by using the code that is listed according to:
php artisan make:model Product -m
Then, upgrade your file to include the required field. Add title and description fields for the product model and these two table fields inside the database/migrations/date_stamp_create_products_table.php file.
$table->string('title'); $table->longText('description');
The next step is to make those fields filled to the max. Inside app/Models/Product.php, make title
and description
fillable fields.
protected $fillable = ['title', 'description'];
What are the things you should be aware of about the Controller?
Develop a controller which can control the device using the command. This will create the app/Http/Controllers/Api/ProductController.php file.
php artisan make:controller Api\\ProductController --model=Product
Develop the logic to create and retrieve objects. Within an index index
method, you can incorporate this code to take a examine every object.
products = $products x all :(); Return the result ()->json(["status" is"false" as well "products" exceeds"$products");
Then, you need to include a StoreProductRequest
class to store new items inside the database. This class will be placed on top of the existing file.
public function store(StoreProductRequest $request) $product = Product::create($request->all()); return response()->json([ 'status' => true, 'message' => "Product Created successfully! ", 'product' => $product ], 200);
Following procedure is to begin the process of making the request. It is done using this command:
php artisan make:request StoreProductRequest
If you want to add validations, you can use the app/Http/Requests/StoreProductRequest.php file. It's a test that doesn't have validation.
What Are You Required To Create A Route
The last step before you attempt with an API is to design the brand new route. For this, include this code within the routes/api.php file. The file should contain the usage
declaration at the top of the file. Additionally, you should include the route
assertion in the body
use App\Http\Controllers\Api\ProductController; Route::apiResource('products', ProductController::class);
Before you start testing the API, be sure there is an products table exists within your database. If it is not there make it with the aid of a control panel, such as XAMPP. Additionally, you could make use of the following commands to move the table
php artisan migrate
Which is the best way to test an API? API
Before testing the API, ensure that the authorize
method inside the app/Http/Requests/StoreProductRequest.php is set to return true
.
Make a brand new product using Postman. Start by hitting a POST
request to this URL: http://127.0.0.1:8000/api/products/. As this is an POST request for an
application that will facilitate the creation of a new product, it's essential to supply an JSON object with the description and name.
"title":"Apple", "description":"Best Apples of the world"
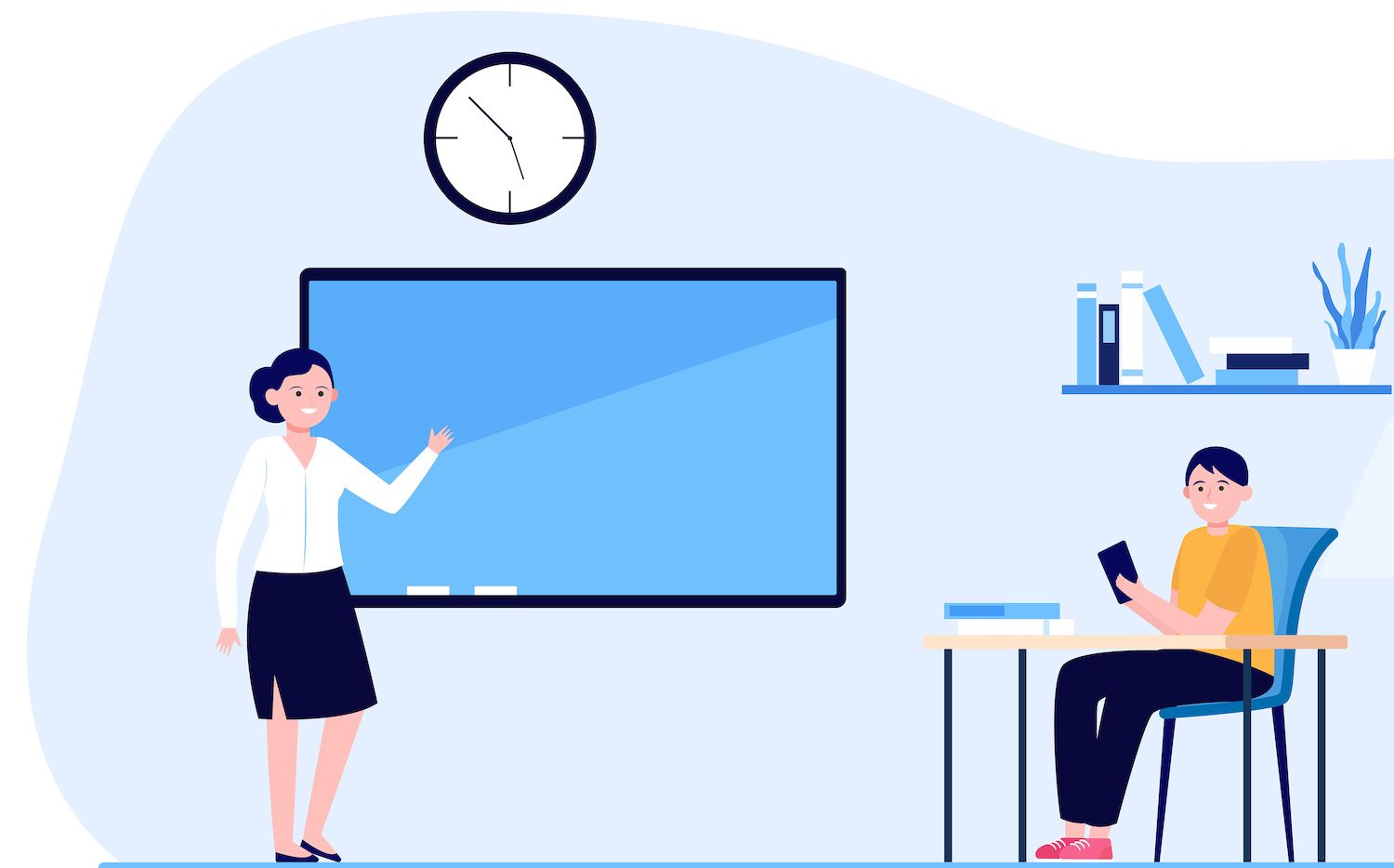
Once you've clicked"Send" when you've clicked"Send" after you've clicked the "Send" button You'll receive the following details:
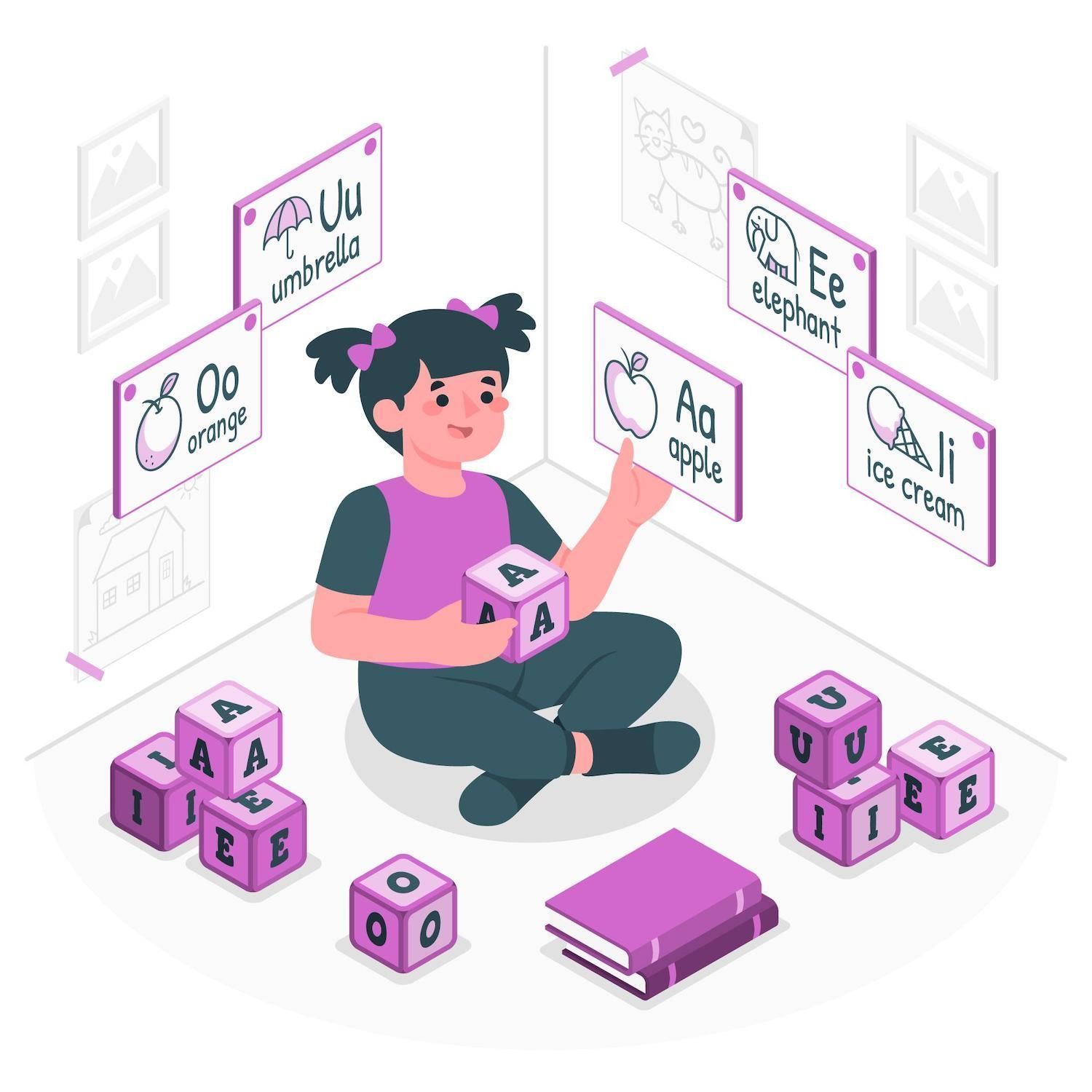
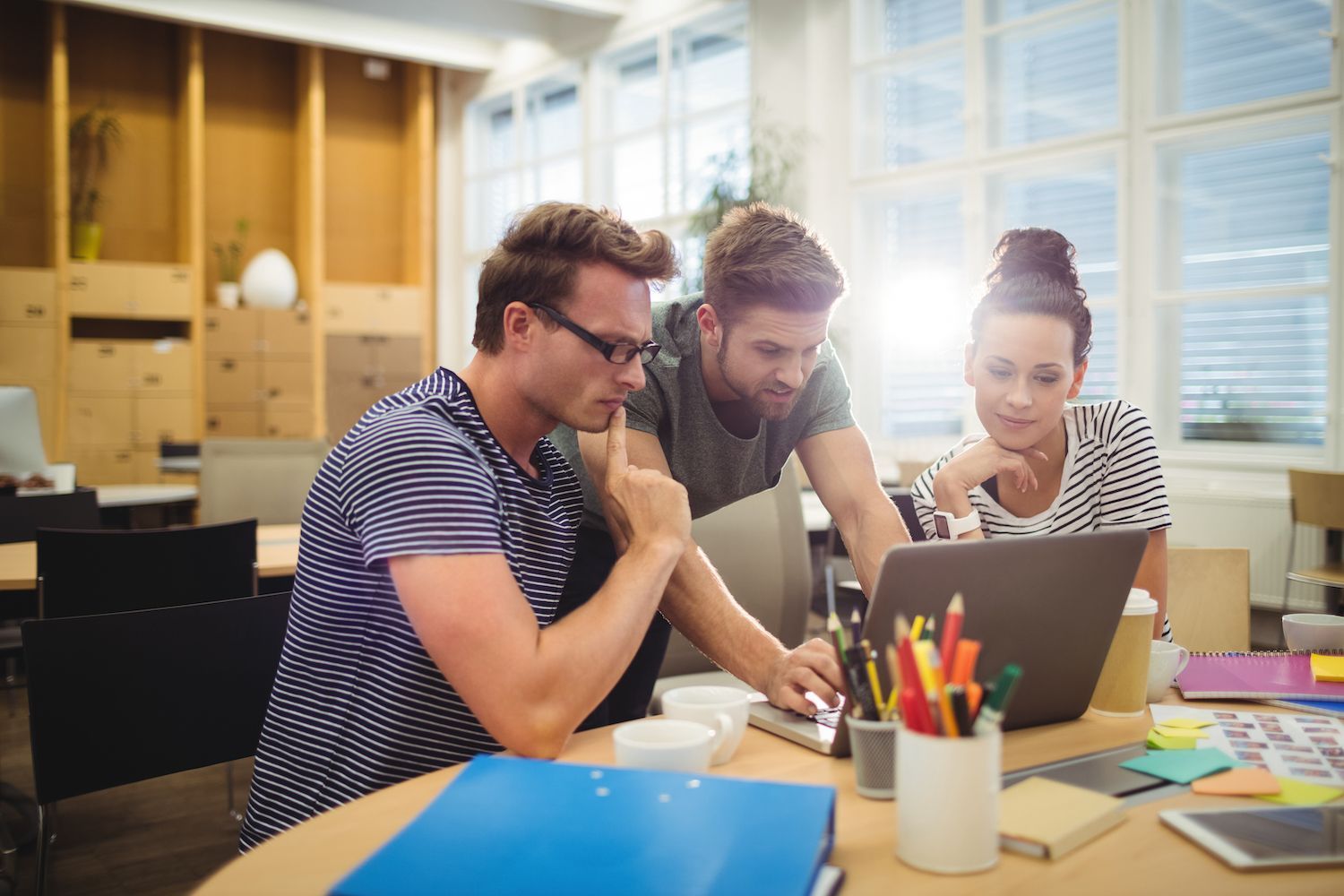
Which is the most efficient method to authenticate APIs with Sanctum?
Security of your API depends on the authentication. your API. Laravel aids in this process through the security features which is part of the Sanctum token. It is a kind of intermediaryware. It encrypts APIs using tokens that are created whenever users sign into their accounts by using the correct username and login. You must be aware that a user will have no access to the API that is secured with tokens.
The first step for adding authentication is to add the Sanctum package with the below code:
composer require laravel/sanctum
Make sure you have your Sanctum Configuration file
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
You can then include the token from Sanctum into middleware. Within in your app/Http/Kernel.php file, it is recommended to apply the following classes and replace middlewareGroups
by using the code below to connect to the middleware group's API.
use Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful;
protected $middlewareGroups = [ 'web' => [ \App\Http\Middleware\EncryptCookies::class, \Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class, \Illuminate\Session\Middleware\StartSession::class, // \Illuminate\Session\Middleware\AuthenticateSession::class, \Illuminate\View\Middleware\ShareErrorsFromSession::class, \App\Http\Middleware\VerifyCsrfToken::class, \Illuminate\Routing\Middleware\SubstituteBindings::class, ], 'api' => [ EnsureFrontendRequestsAreStateful::class, 'throttle:api', \Illuminate\Routing\Middleware\SubstituteBindings::class, ], ];
The next stage is to develop a UserController. UserController
is then added to an application code to create an authentication token.
php artisan make:controller UserController
After creating the UserController
, navigate to the app/Http/Controllers/UserController.php file and replace the existing code with the following code:
Before attempting to authenticate, it is essential to create an account for an individual user with seeders. The command will generate the users table document.
php artisan make:seeder UsersTableSeeder
Inside the database/seeders/UsersTableSeeder.php file, replace the existing code with the following code to seed the user: insert([ 'name' => 'John Doe', 'email' => '[email protected]', 'password' => Hash::make('password') ]);
Begin now to implement this guideline:
php artisan db:seed --class=UsersTableSeeder
The last step in the authentication procedure is to install the middleware designed to secure the routing. Check the routes/api.php file, and then add the routing for your product in it. routes/api.php file and incorporate the route you have created from your product in middleware.
use App\Http\Controllers\UserController; Route::group(['middleware' => 'auth:sanctum'], function () Route::apiResource('products', ProductController::class); ); Route::post("login",[UserController::class,'index']);
If you've established a brand new route by using the middleware you'll see an error message coming in the internals of your server whenever you attempt to download the software.
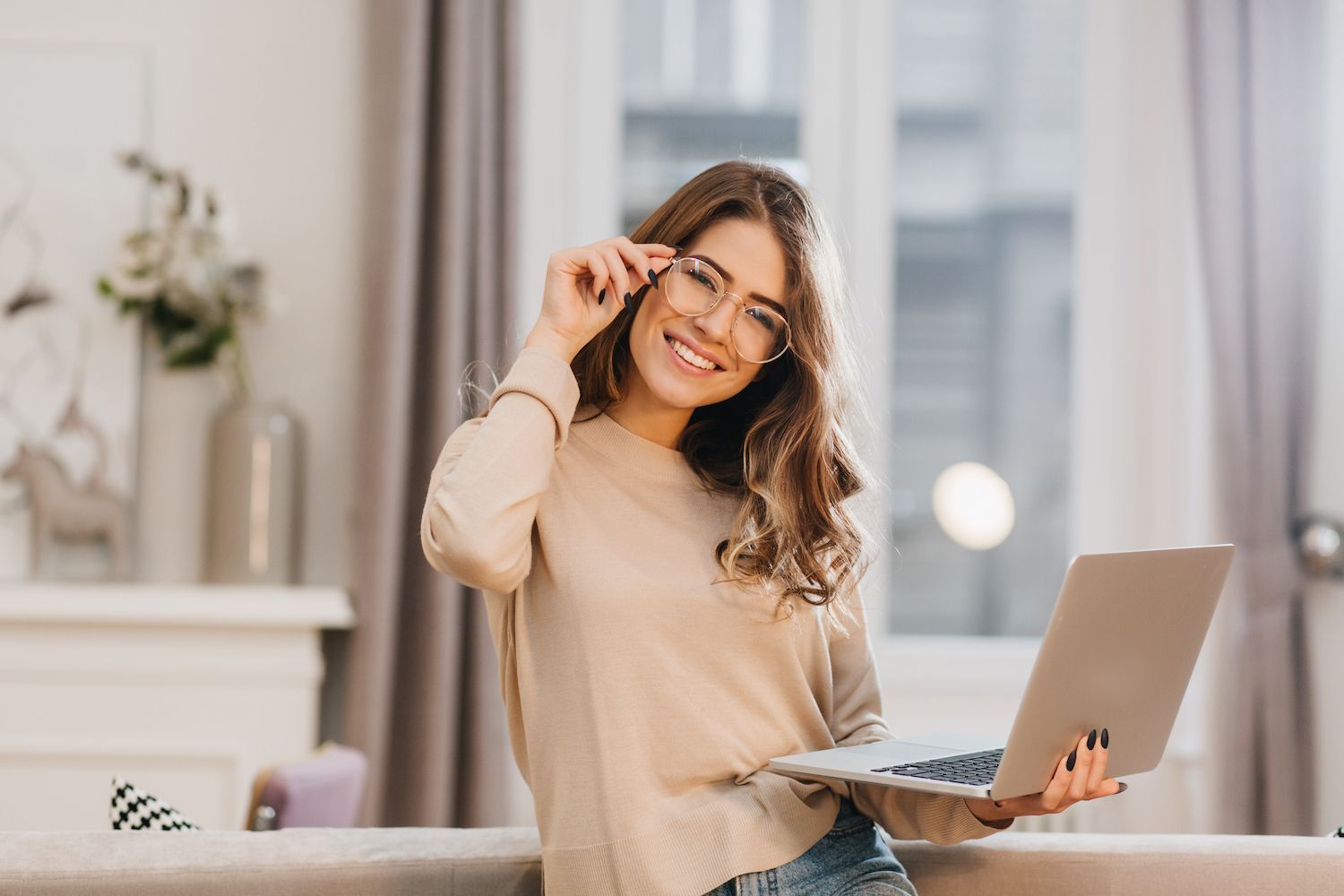
When you've successfully connected with your username, you'll receive personal tokens to usage with your account. If you decide to use it, make sure to include the HTTP header. It will then verify your identity before working. You can send a POST request to http://127.0.0.1:8000/api/login with the following body:
"email":"[email protected]", "password":"password"
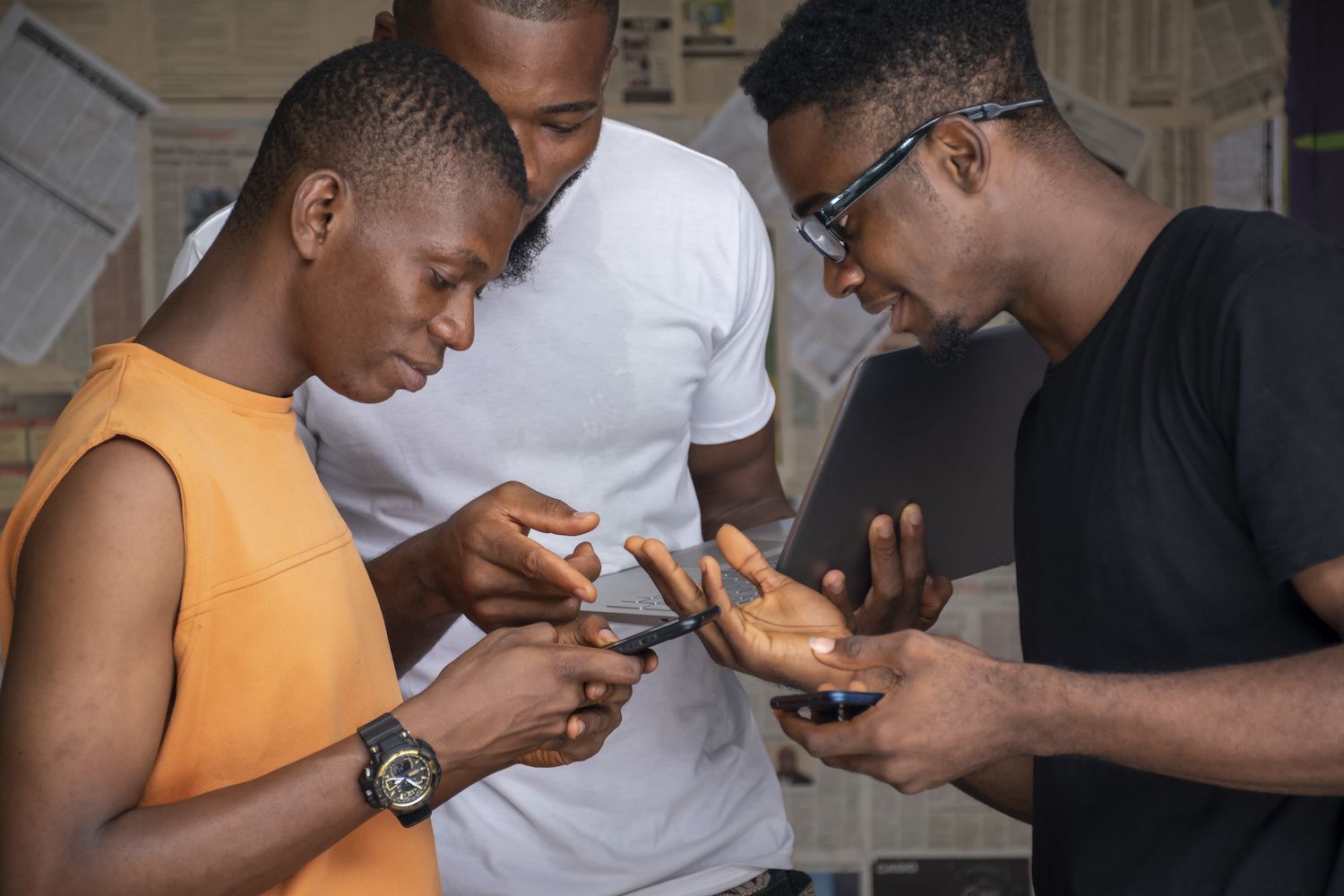
Use the token you received as a bearer token, and then include it in the header of Authorization.
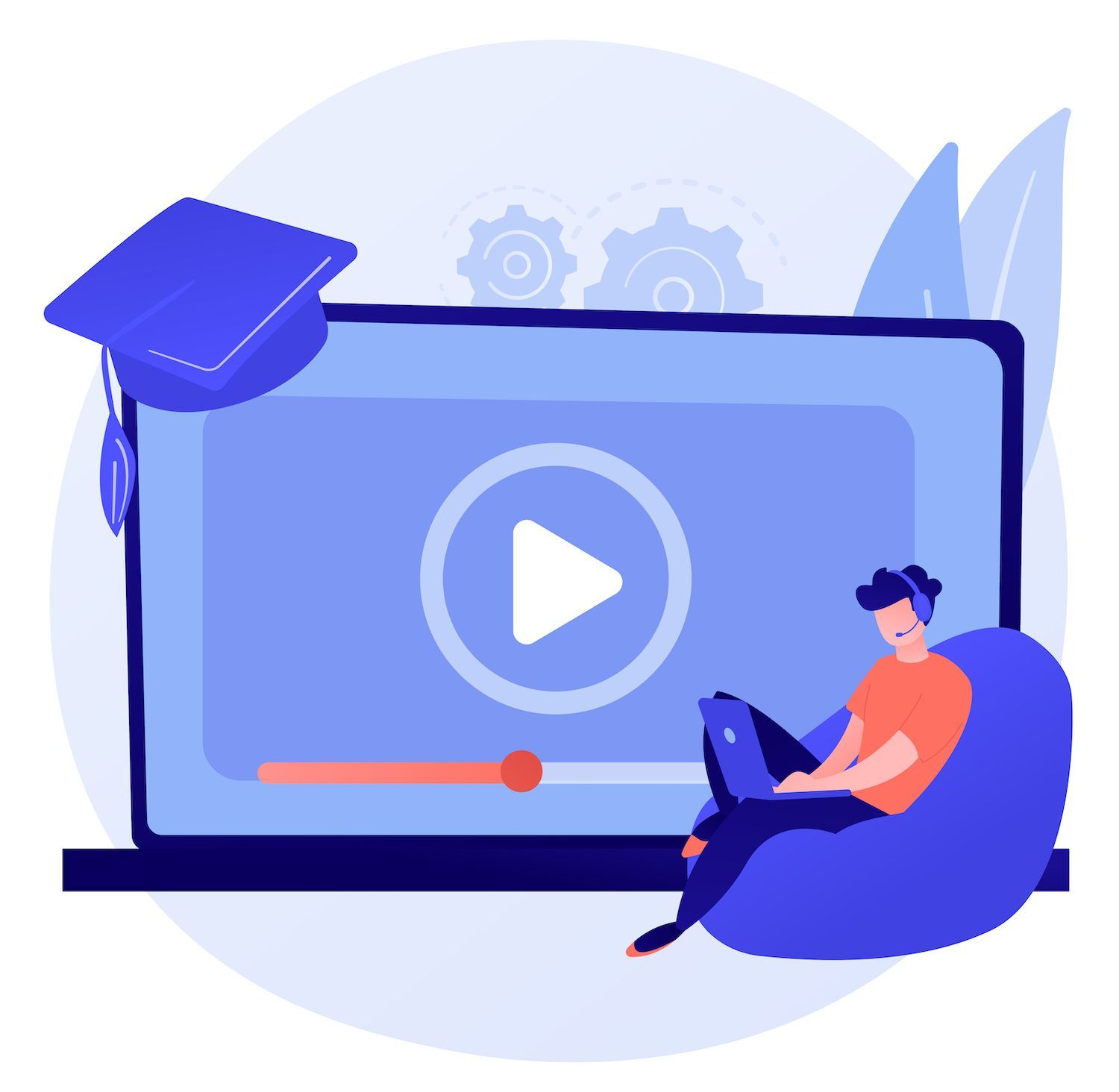
What are you able to accomplish to fix API Errors?
An error code by itself will not be enough. A message for error that can be easily read by a person is essential. Laravel offers a variety of choices to address the problem. Utilize a fallback, catch block, or an individual response. The code below you paste in the UserController UserController
will demonstrate this.
if (!$user || !Hash::check($request->password, $user->password)) return response([ 'message' => ['These credentials do not match our records.'] ], 404);
Summary
The Laravel Eloquent Model makes it easy to design and develop the API and then test it. Mapping objects offers the ability to seamlessly connect to databases.
In addition, it acts as a middleware. The token Sanctum created by Laravel assists in protecting APIs in a very short time.
- My dashboard is easy to control and configure inside My dashboard. My dashboard
- Support is available 24 hours a day.
- The most secure Google Cloud Platform hardware and network is powered by Kubernetes to improve the performance.
- Premium Cloudflare integration that speeds the process as well as improve security
- Global reach with more than 35 centers for data as plus 227 points of contact around the globe
The original article appeared on this website
The article appeared on here
This post was first seen on here
This post was posted on here