Create a -Hosted To-Do List Using Jira API & React
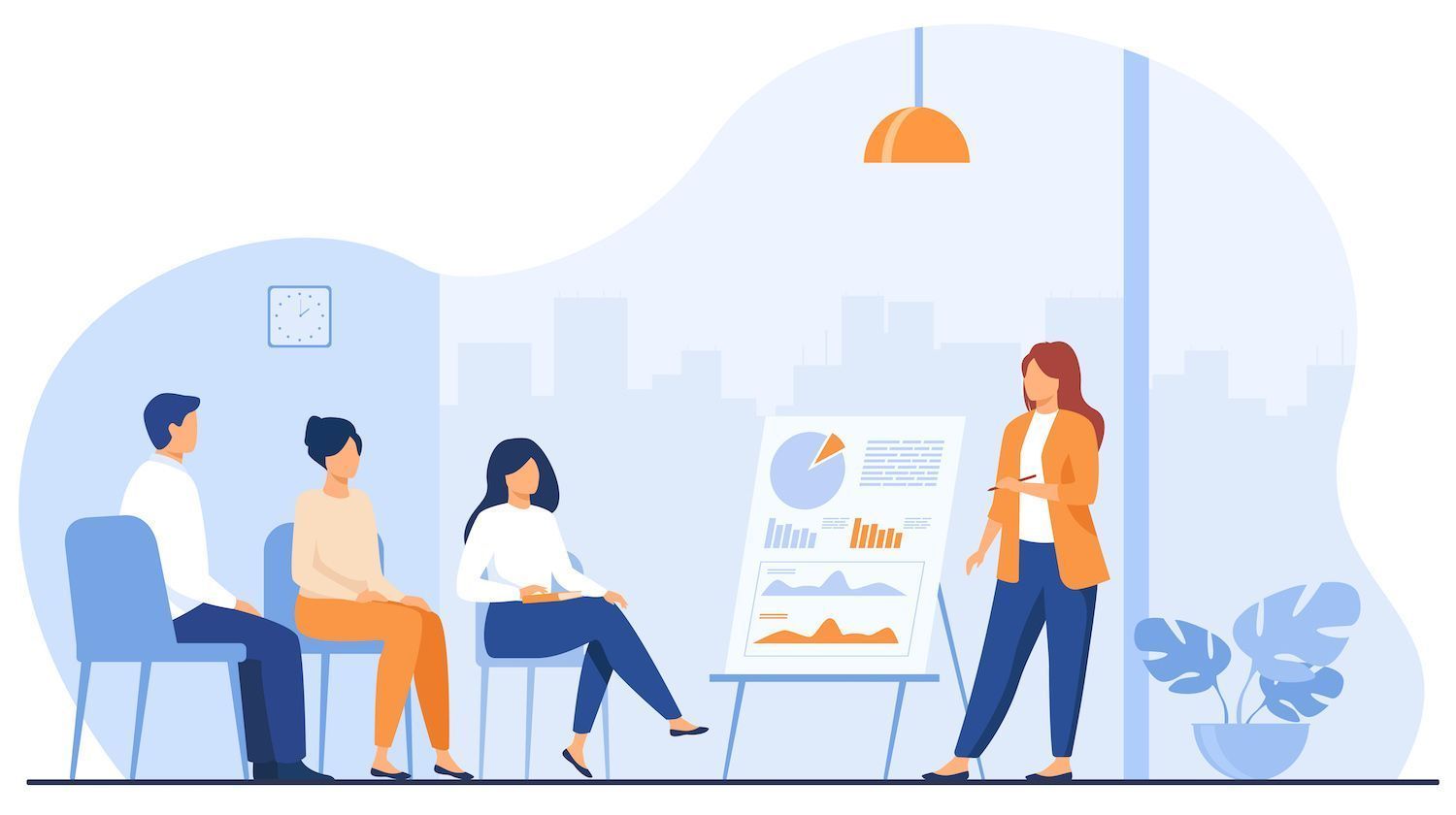
-sidebar-toc>
Jira is an extremely well-known program to manage projects, which lets you manage the projects' tasks. If you're involved with an immense project it is likely that the Jira dashboard could become overwhelmed due to the sheer volume of tasks. Additionally, the teams are more numerous.
To resolve the issue, for this issue to be solved, you can use Jira to solve this problem, utilize Jira's Jira REST API to build a basic To-Do list application which lists your tasks that you must finish along with dates. It lets you programmedly interact with Jira for building, access the latest updates, remove problems or make them, as well look up information on the people that you've contacted, and also information about the application you've created.
The rules
In order for you to be able to comply with the guidelines for adhering to
- Node.js and the Node Package Manager (npm) can be installed on your system to help you develop.
- An Jira account that has an account on Jira that lets you see the project.
How do I best to build the backend using Node.js and Express
These steps will help you to establish your server.
- Create a brand-new directory and then go to it. After that, you'll be ready to start Node.js by following these steps:
NPM init"y"
This command is used to create a package.json file with default settings. These files is stored in the directory that is the root of the directory used by the program.
- Then, you must complete the dependencies essential to complete your project using the following command line:
NPM install express dotenv Axios
This command will set up the following
express
is a basic Node.js framework that allows developers to create APIs.- dotenv is a piece of software that is loaded through
.env
variables toprocess.env
to let you access them securely. Axios
is an promise-based HTTP client that was specifically developed to be compatible with Node.js. It was developed to permit API requests to Jira.
- If your installation succeeded If it did, you need to you can create an.env file. .env The file should be placed at the root of the project. It is necessary to enter the
number of the port
number:
PORT=3000
The port number determines which port the server is listening to. You can alter it to any port that you want.
- Create index.js. index.js The file can be found in that directory. It is possible to add the following code to load Express in your project. It is necessary to start by using a program named Express. Launch Express. Open the Express application. Open your server
const express = require('express'); require('dotenv').config() const app = express(); const PORT = process.env.PORT; // Define routes here app.listen(PORT, () => console.log(`Server is running on port $PORT`); );
- If you're able complete the process step and include your package.json File, add a script that will start the server.
"scripts": "start": "node index" ,
Then, you're able to run the script using the terminal that you've been using.
NPM run begin
This command is used to start your server. This message will be displayed in the terminal.
Server is running at 3000 ports.
If your server is running functioning after the server is fully operational, it will be in a situation to establish options for Jira. Jira application.
How do I configure an Jira App
For Jira REST API to be used to access Jira REST API, you need to join the Jira site. Jira REST API is available to use to it, you need to sign up as an user on the Jira website. The API that Jira is in development and relies on easy authentication process using the Atlassian Account email and API token.
The way to setup it:
- Sign up for your own Jira account or sign-in if you already have an account.
- You can join the security tab within the security tab on the security tab of your Atlassian profile. Click the option that will create API tokens..
- When the dialog opens the user is required to provide a primary name for the item you wish to save (e.g., "jira-todo-list") and then press the Create button..
- Transfer the token onto your clipboard.
- After saving the API token to the file .env file:
JIRA_API_TOKEN="your-api-token"
Now, you can utilize Jira API. Jira API with the basic authentication.
Discover ways to recover problems from Jira
After you've set up your Jira application. Design the routes that will create issues for Jira inside the framework within your Node.js server.
To submit an API request using Jira API You must first connect to Jira API to utilize your Jira API token which you have saved in the .env file. The API token is saved in process.env
and assign it to the variable JIRA_API_TOKEN
within your index.js file. index.js file:
const JIRA_API_TOKEN = process.env.JIRA_API_TOKEN
The next step is to add the URL of your website to API endpoint. API request. The URL is comprised of the Jira domain and the Jira query Language (JQL) assertion. The Jira domain acts as an URL that represents the identity of your Jira business and is similar to org.atlassian.net
, where it is the phrase "org"
is the corporate name of your business. JQL is a different matter. JQL is a query-oriented programming language that allows you to communicate for the purpose of solving problems by using Jira.
First, you must add your Jira domain to the.env file. .env file:
JIRA_DOMAIN="your-jira-domain"
Also, it is essential to store your Jira email within the.env file. .env file as it's used to obtain the authentication required to forward your Jira application:
JIRA_EMAIL="your-jira-email"
You'll then need to make two environment variables. Then, you'll have the ability to create the URL of your site with the help of the domain, as well as a JQL query. This query could be utilized to solve issues that involve "In this process" or "To complete" declares the position that the user is. This query can then sort out the problems based on the state of every.
const jiraDomain = process.env.JIRA_DOMAIN; const email= process.env.JIRA_EMAIL; const jql = "status%20in%20(%22In%20progress%22%2C%20%22To%20do%22)%20OR%20assignee%20%3D%20currentUser()%20order%20by%20status"; const apiUrl = `https://$jiraDomain/rest/api/3/search?jql=$jql`;
When creating a new route, it is necessary to incorporate Axios inside the index.js file.
const axios = require("axios")
Now you can design your own route, then send an GET request to the Jira API. Jira API. Jira API to return questions. Index.js should contain the below code: index.js include the following instructions: index.js add the below code:
app.get('/issues/all', async (req, res) => )
Use using the axios.get
method to generate a GET call to Jira's Jira REST API. You can create the Authorization. Authorization
header, which utilizes the base64 encryption method to safeguard your Jira email. It also functions as serving as an API token
const response = await axios.get(apiUrl, headers: Authorization: `Basic $Buffer.from( `$email:$JIRA_API_TOKEN` ).toString("base64")`, Accept: "application/json", , );
Get the response from Jira API. Jira API. Jira API will store the response as an array of variables. The response will include property information about problems
as well as a list of issues objects:
cont. data = Expect response.json() Problems with const relate to the result of data
Then, you'll go through the question
list, and remove all relevant information about the work to be done You'll then return to it by submitting the JSON response. JSON response.
let cleanedIssues = []; issues.forEach((issue) => const issueData = id: issue.id, projectName: issue.fields.project.name, status: issue.fields.status.name, deadline: issue.fields.duedate, ; cleanedIssues.push(issueData); ); res.status(200).json( issues: cleanedIssues );
If you make a request to http://localhost:3000/issues/all
, you should receive a JSON object containing the issues:
curl localhost:3000/issues/all
It is possible to take it to the next level through the assistance of companies like SendGrid as well as the cron task which will mail out daily messages according to the jobs that you've set.
Host Your Node.js App to
- Installation of the cors npm software with the following command in your terminal:
Cors have now been included in NPM
- Once you've uploaded the file, it's going to be open, and then you'll be able to choose including index.js in the folder. index.js .
Const Cors = require('cors')
- It is then feasible to build CORS that serve as a middleware. It can let it respond to every request. The following code should be added at the beginning of index.js file. index.js file. index.js file:
app.use(cors());
It is now possible to send HTTP request to the server by using an alternate domain. This way, you'll know that you aren't experiencing CORS error messages.
- Register or log in to your account to gain access to your dashboard. My dashboard.
- It is necessary to authorize your Git service provider.
- Choose the correct programs from the menu on the left. Select the appropriate programs. Click on the Add button..
- Choose the repository you'd like to create and then select the branch that you'd like to make available from.
- Pick a unique branding name for your app and pick an appropriate site for the data center.
- Include your environment variables. There's nothing wrong with making the PORT a variable in your environment because this is a process that happens automatically. Look for the checkboxes that are close to the time of running and accessible during the build process :
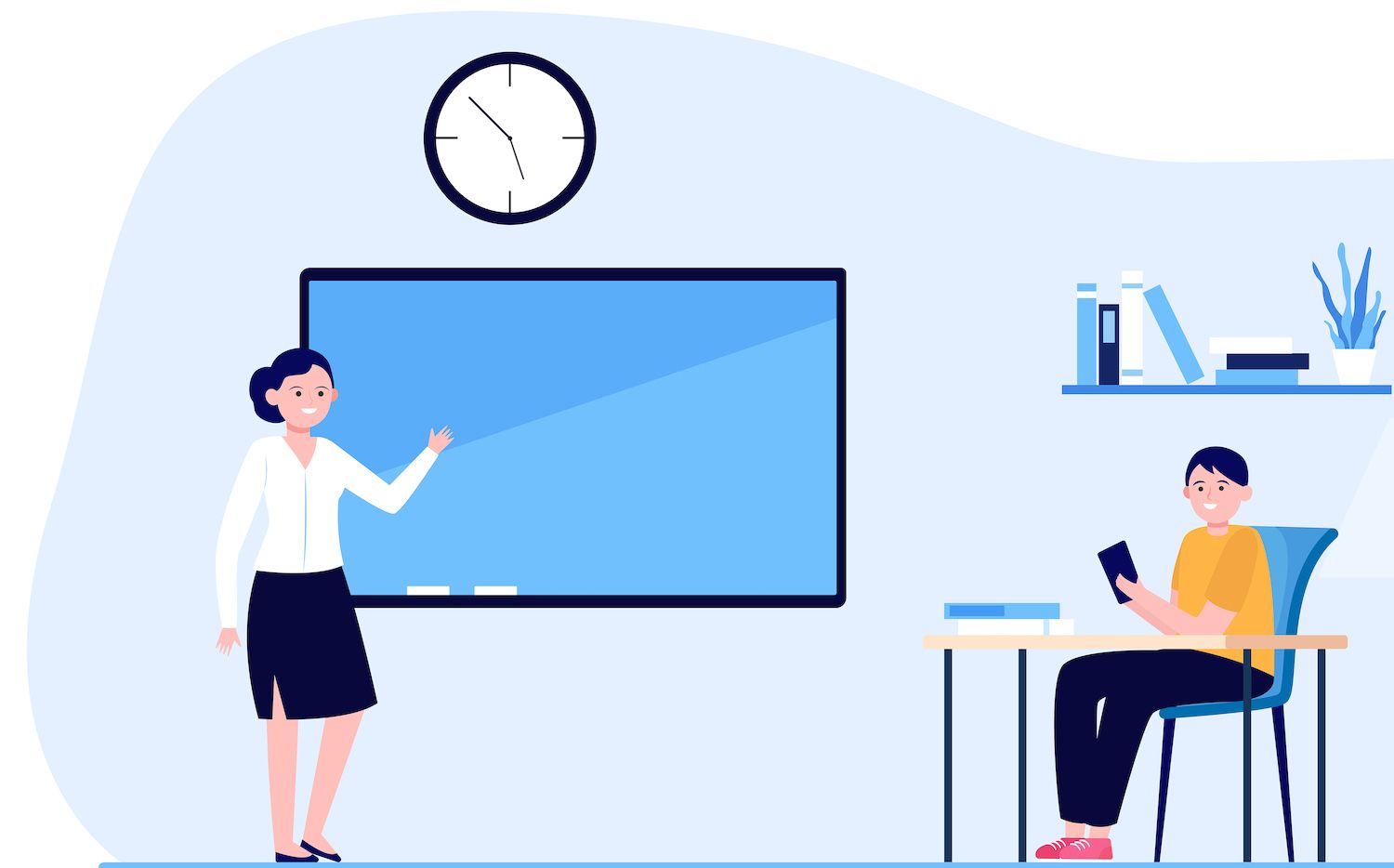
- Check other information (you may change your default settings) after which click to sign up for your account..
The server is successfully installed . From the menu on the left, choose Domains and take the domain you wish to make your main. This is the address of your server's endpoint.
Create an React application that demonstrates the problems
- A fresh React project called
jira-todo
:
npx create-vite jira-todo --template react
- Go to the directory of your project and install any dependencies are required:
Installation NPM
- Set up a server that allows development
npm run dev
There are issues with retrieving the data from the server
- Remove the contents from App.jsx and add the following code:
Function program() *, which is the application program set to default to export details
- Before you start to notice problems, you need to create the URL for the server in your .env file at the root of your program's directory:
VITE_SERVER_BASE_URL="your-hosted-url"
- Look for the URL within App.jsx by adding this line at the very at the top of the file:
const SERVER_BASE_URL=import.meta.env.VITE_SERVER_BASE_URL
- In your component, you'll be required to develop an async program that's named
fetchData
after which you'll need to make an HTTP request to anissue/all
endpoint of the Express server. If you are able to obtain an answer, you must be able to transform it to JSON and save the data as a number that is known as "data. ":
import useCallback, useEffect, useState from "react"; function App() const SERVER_BASE_URL=import.meta.env.VITE_SERVER_BASE_URL const [data, setData] = useState([]); const fetchData = useCallback(async () => try const response = await fetch(`$SERVER_BASE_URL/issues/all`); if (!response.ok) throw new Error('Network response was not ok'); const data = await response.json(); setData(data.issues); catch (error) console.error('Error fetching data:', error); ,[SERVER_BASE_URL]); useEffect(() => // Fetch data when the component mounts fetchData(); ,[fetchData]); return ( What's on my list today? ); export default App;
Use use of your utilizeEffect
hook to execute fetchData. fetchData
function will be executed once the component is beginning to expand in dimension.
Show the problems from Jira in the browser for web.
- You are then in a position to change your return messages from the program so that it can run through the issues and show messages on the web browser.
return ( What is the first task on my agenda of things to accomplish this day? data && data.map(issue => return issue.summary issue.deadline className="status">issue.status ) );
- For styling this application the look, simply add the below CSS code into App.css:
h1 text-align: center; font-size: 1.6rem; margin-top: 1rem; section display: flex; flex-direction: column; justify-content: center; align-items: center; margin-top: 2rem; .issues display: flex; min-width: 350px; justify-content: space-between; padding: 1rem; background-color: #eee; margin-bottom: 1rem; small color: gray; .status-btn padding: 4px; border: 1px solid #000; border-radius: 5px;
- After that after that, then, load App.css in index.js to apply the styles:
import './App.css'
If you launch your application and then launch your application and then launch it, you'll start seeing the assignments you've received and the dates that they were completed, as well as the status of your web browser.
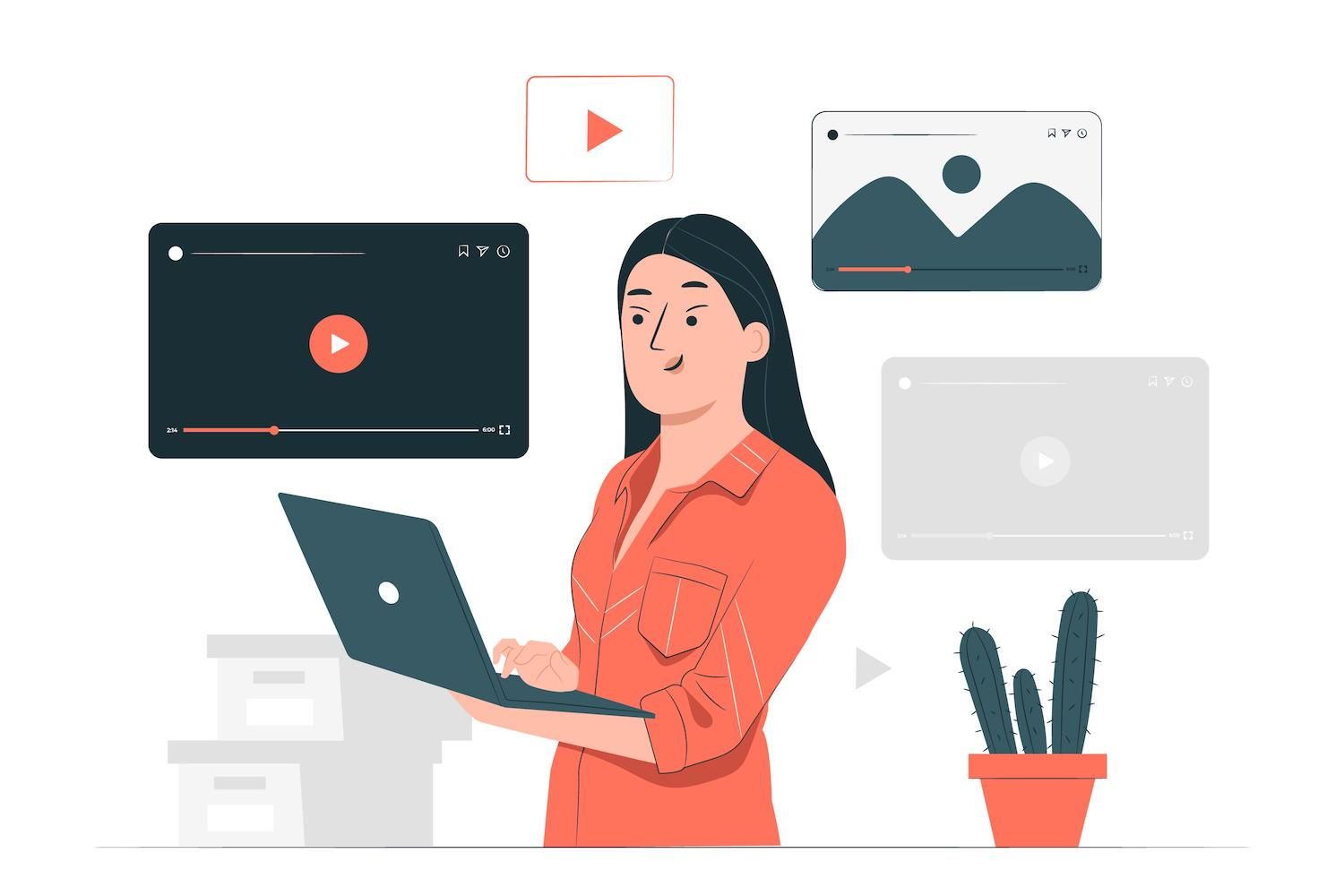
The application can be deployed to be installed on
- Sign up or sign in to your account to get access to My Dashboard. My dashboard.
- You need to authorize Git to connect with your existing services.
- Choose static sites in the left-hand sidebar. Then, select Add Site. Select to Add Site..
- Select the repository you'd like to deploy from, along with the branch that you'd like to deploy from.
- Assign a unique name to your site.
- My detects the setting for building that comprise React Project automatically. React Project automatically. The settings are already filled into:
- Builder command
NPM run build
- Node version:
18.16.0
- Publish directory:
dist
- Add the URL of your server as an environment variable using
VITE_SERVER_BASE_URL
. - Once you've finished the steps above, you'll have the ability to hit on the link to start your website..
Summary
In this tutorial for beginners, you'll discover how you can create an Express application that can retrieve well-known Jira issues using Jira's Jira REST API. In addition, you included the React frontend of your Express application to show the problems on the browser.
This app offers a simple illustration of what you can achieve using Jira's REST API. It is possible to enhance the app with functions that enable you to keep notes of your tasks that you've completed and also perform sophisticated filters, as well as many more.
Jeremy Holcombe
Editorial Editor as well as Editorial Editor as well as WordPress Web Developer as well as Content Writer. Apart from everything connected to WordPress I love the beach golf, beaches and beaches and watching films. Additionally, I suffer from difficulties with height ;).
The first time the post was posted, it appeared this website
The article was published on this website
This post was posted on this website.
This post was first seen on here