Create a -Hosted To-Do List Using Jira API & React
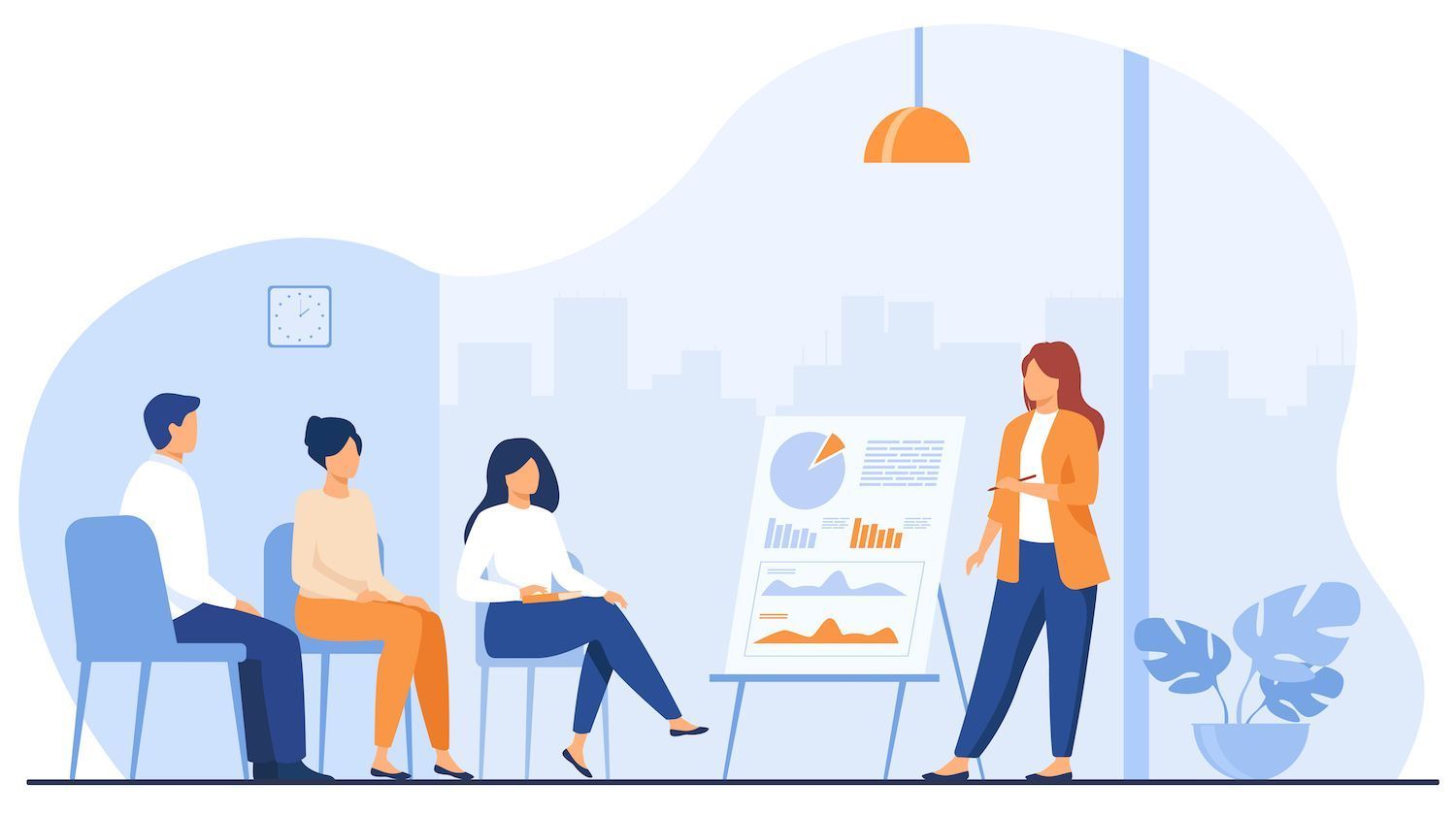
-sidebar-toc>
Jira is a well-known software for managing projects that lets you manage projects' tasks. If you're involved in an enormous project, the Jira dashboard can become overloaded due to the huge quantity of work. Also, the team members increase.
To address the issue, for this problem to be addressed, use to address this issue, you can use Jira's Jira REST API to develop a straightforward To-Do List application that displays the tasks you have to be able to complete, as well as deadlines. The API lets you programmatically interact with Jira to build, access the latest updates, remove problems or even create them and as well view information about the users you've contacted and information about your application.
The requirements
For you to be able to comply with the guidelines for following along
- Node.js and Node Package Manager (npm) are installed on your system for development.
- A Jira account that has a Jira that lets you see the projects.
How do I best to create the backend Utilizing Node.js as well as Express
These steps will help you set up your server
- Create a new directory and go to it. After that, you'll be in a position to launch Node.js by following these steps:
NPM init"y"
This command can create the package.json file with default settings that are located within the root directory of the directory of the application.
- You must then add the necessary dependencies for your project using the below command line:
NPM install express dotenv Axios
The command that follows installs following:
express
is a very simple Node.js framework that lets you to create APIs.- dotenv is a software program that is loaded through
.env
variables toprocess.env
to let you securely access them. Axios
is a promise-based HTTP client that was designed specifically to work with Node.js. It is built to permit API calls to Jira.
- If the installation was successful, create an.env file. .env The file is required to be in the root your project. Enter the
port
number:
PORT=3000
The port number determines the port that the server is listening to. You can alter it to any port that you want.
- Make index.js. index.js The file lies within the root directory. You can add the following code to load Express to your project. Start with an application called Express. Open the Express application. Open your server
const express = require('express'); require('dotenv').config() const app = express(); const PORT = process.env.PORT; // Define routes here app.listen(PORT, () => console.log(`Server is running on port $PORT`); );
- If you are able to finish the job step, add in the package.json File, include a script to start the server.
"scripts": "start": "node index" ,
Now you can execute your script via the terminal that you're using.
NPM run begin
This command starts your server. The following message in your terminal.
Server is running at 3000 port.
If the server is up and operating After the server has been up and operational, you'll be in a position to set up options for the Jira application.
How to configure an Jira App
To access the Jira REST API for access, you must sign up as an account user on your Jira website. The app API that your application develops relies on a simple authentication via your Atlassian Account email and API token.
The way to set it up:
- Make a Jira login or sign in when there's already an account.
- Connect to the security tab within your Atlassian profile. Then, choose the button that will create an the API token..
- In the dialogue that opens the user is required to type in an initial name for the thing you wish to record (e.g., "jira-todo-list") then hit the Create button..
- Copy the token to your clipboard.
- Once you have saved the API token in your .env file:
JIRA_API_TOKEN="your-api-token"
Now, you can utilize Jira API. Jira API with the basic authentication.
Find routes to retrieve problems from Jira
Once you've setup the Jira application. Set up the routes that bring issues to Jira within your Node.js server.
For you to make an API request using Jira API, you must first access Jira API, you must make use of your Jira API token you saved in your .env file. You can locate the API token using process.env
and assign it to the variable JIRA_API_TOKEN
within the index.js file. index.js file:
const JIRA_API_TOKEN = process.env.JIRA_API_TOKEN
The next step is to add the URL for your API endpoint. API request. The URL is made up of the Jira domain as well as the Jira query Language (JQL) assertion. The Jira domain acts as an URL for your Jira organization and is akin to org.atlassian.net
, where the term "org"
is the name of your business. JQL However, JQL is a query-oriented language that is used to communicate to solve the issues of Jira.
The first step is to include your Jira domain into the.env file: .env file:
JIRA_DOMAIN="your-jira-domain"
It's also essential to save your Jira email in the.env file. .env file as it's going to be used in the authorization necessary to send your application Jira:
JIRA_EMAIL="your-jira-email"
After that, you need to create two environment variables. Following that, you're able to make the URL for your destination using the domain as well as the following JQL query. This query can be used to sort out issues with "In this process" or "To complete" statuses that the client is in. Then, it sorts the issues based on the status of each.
const jiraDomain = process.env.JIRA_DOMAIN; const email= process.env.JIRA_EMAIL; const jql = "status%20in%20(%22In%20progress%22%2C%20%22To%20do%22)%20OR%20assignee%20%3D%20currentUser()%20order%20by%20status"; const apiUrl = `https://$jiraDomain/rest/api/3/search?jql=$jql`;
Before creating a route, it is essential to include Axios into the index.js file.
const axios = require("axios")
You can now create an entirely new route and send an GET request to the Jira API. Jira API to return questions. In index.js include the following code: index.js add the below code:
app.get('/issues/all', async (req, res) => )
Make use of the axios.get
method to create a GET call to Jira's Jira REST API. Make an Authorization header. authorization
header, which utilizes the base64 encryption to protect your Jira email, as well as an API token
const response = await axios.get(apiUrl, headers: Authorization: `Basic $Buffer.from( `$email:$JIRA_API_TOKEN` ).toString("base64")`, Accept: "application/json", , );
Seek out the response from the Jira API. Jira API and store it in an array of variable. The response will include properties issues
and a list of issues objects:
cont. data = expect response.json() Issues with const are data
You'll then go through the the questions
listing, and remove only the relevant information about the tasks to be completed, and then return to it in JSON response. JSON response.
let cleanedIssues = []; issues.forEach((issue) => const issueData = id: issue.id, projectName: issue.fields.project.name, status: issue.fields.status.name, deadline: issue.fields.duedate, ; cleanedIssues.push(issueData); ); res.status(200).json( issues: cleanedIssues );
If you make a request to http://localhost:3000/issues/all
, you should receive a JSON object containing the issues:
curl localhost:3000/issues/all
It can be elevated to the next level with the help of a company like SendGrid as well as the cron task which will send out daily emails according to the tasks you have assigned.
Host Your Node.js App to
- Installation of the cors npm program by running the following command from your terminal:
adding cors to NPM
- After you've imported your package, it can be open, and then you'll be able to add index.js in the file. index.js .
Const Cors = require('cors')
- It is then possible to create CORS to function as a middleware and allow it to respond to all requests. The following code should be added at the beginning of the index.js file index.js file:
app.use(cors());
Now you can send HTTP requests to the server using an alternate domain, so you can rest certain that you don't experience CORS errors.
- Sign up or sign in to your account in order to gain access to the dashboard of your My dashboard.
- It is necessary to authorize your Git service supplier.
- Select the appropriate applications from the left sidebar. Click on on the Add button..
- Choose the repository you want to make available and then select the branch you would like to make available from.
- Choose a distinct branding tag for your app and pick an appropriate data center site.
- Include your environment variables. There's no reason to make the PORT an environment variable because the process is carried out automatically. Look for the checkboxes next to the time of running and available during the build process :
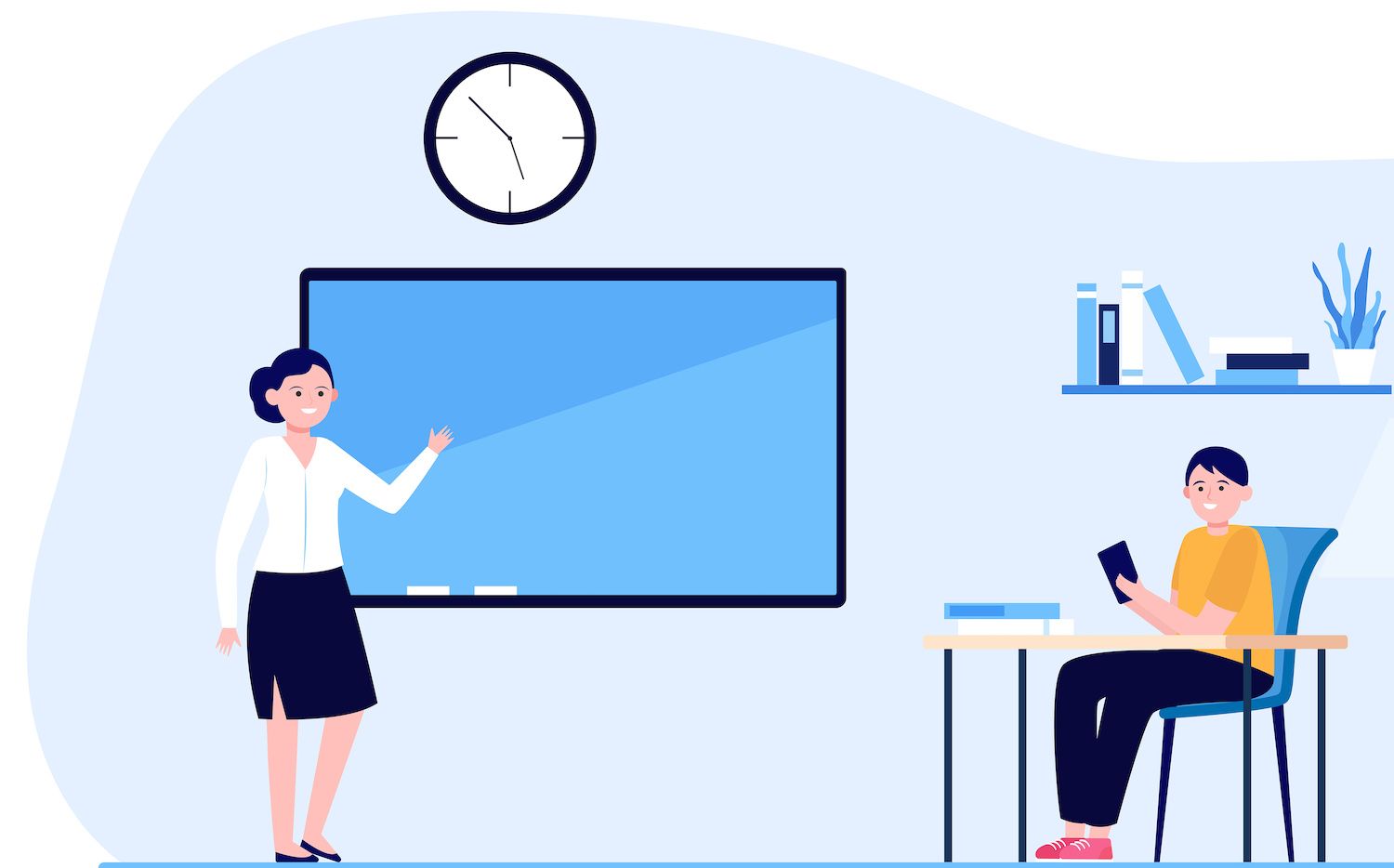
- Examine other data (you may change the defaults) after which click to create an account.
The server is successfully deployed . From the menu on the left, choose Domains and then copy the domain you wish to use as your primary. This will be the URL of your server's endpoint.
Make an React application to demonstrate the difficulties
- A fresh React project called
jira-todo
:
npx create-vite jira-todo --template react
- Go to the directory of your project, and then install all the dependencies that you need:
Installation NPM
- Start the server for development
npm run dev
Problems with fetching information from the server
- Remove the contents of App.jsx and add this code:
function application() *, the application that defaults to export data
- Before you can begin obtaining problems, you need to establish the URL for the server inside a .env file at the root of your application's directory:
VITE_SERVER_BASE_URL="your-hosted-url"
- Get the URL from App.jsx by adding this line at the very top of the file:
const SERVER_BASE_URL=import.meta.env.VITE_SERVER_BASE_URL
- Within your component, you'll need to create an async application that's named
fetchData
and then make an HTTP query for theissues/all
endpoint of the Express server. If you are able to obtain an answer, it's important to convert it into JSON and save the data as a value in the state known as "data. ":
import useCallback, useEffect, useState from "react"; function App() const SERVER_BASE_URL=import.meta.env.VITE_SERVER_BASE_URL const [data, setData] = useState([]); const fetchData = useCallback(async () => try const response = await fetch(`$SERVER_BASE_URL/issues/all`); if (!response.ok) throw new Error('Network response was not ok'); const data = await response.json(); setData(data.issues); catch (error) console.error('Error fetching data:', error); ,[SERVER_BASE_URL]); useEffect(() => // Fetch data when the component mounts fetchData(); ,[fetchData]); return ( What's on my list today? ); export default App;
Make sure you utilize using the useEffect
hook to run fetchData. fetchData
function at the time that your component starts to increase in size.
Show the problems from Jira on the web browser.
- You are then in a position to alter the return statements of the application so it is able to run through the issues and display these on the web browser.
return ( Which item is on my to-do list for the day? data && data.map(issue => return issue.summary issue.deadline className="status">issue.status ) );
- To style this application your way you, simply include the following CSS code to App.css:
h1 text-align: center; font-size: 1.6rem; margin-top: 1rem; section display: flex; flex-direction: column; justify-content: center; align-items: center; margin-top: 2rem; .issues display: flex; min-width: 350px; justify-content: space-between; padding: 1rem; background-color: #eee; margin-bottom: 1rem; small color: gray; .status-btn padding: 4px; border: 1px solid #000; border-radius: 5px;
- Then, import App.css in index.js to apply the styles:
import './App.css'
If you launch your application, you'll be capable of viewing the list of assignments that you've been given, along with the date they were completed and their status in your web browser.
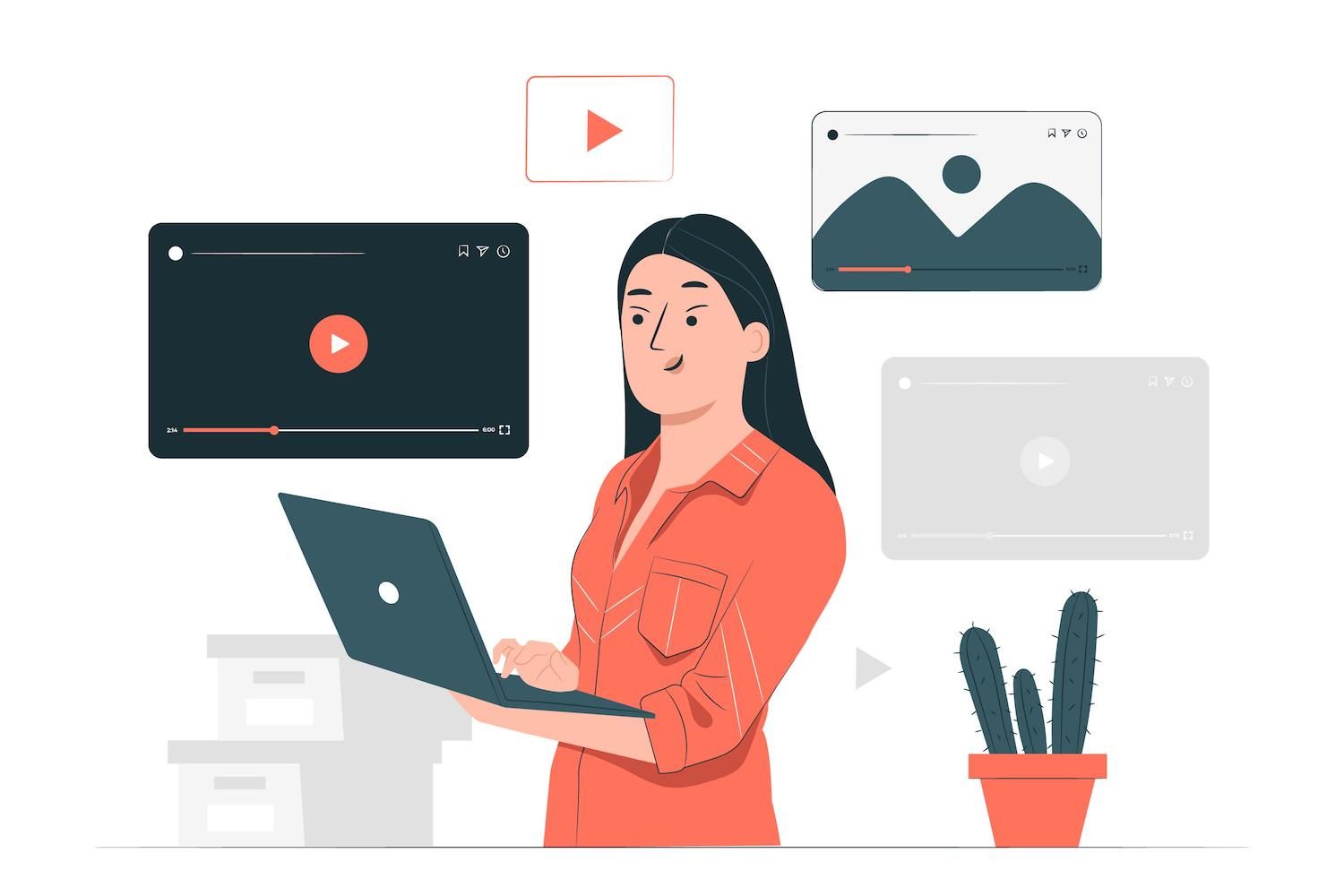
You can deploy the application to be deployed on
- Create or log in to an account to gain access to My dashboard. My dashboard.
- Authorize Git to join with services that you're utilizing.
- Select static sites in the left-hand sidebar. Then click Add Site. Add site.
- Select the repository that you would like to deploy from, along with the branch that you would prefer to deploy from.
- Assign a unique name to your site.
- My is able to detect the settings for building which are part of React Project automatically. React Project automatically. These settings have already been filled in:
- Builder command
NPM run build
- Node version:
18.16.0
- Publish directory:
dist
- Add the URL of your server as an environment variable using
VITE_SERVER_BASE_URL
. - Once you have completed this, you will be in a position to click to create your site..
Summary
In this instructional tutorial, you'll discover how to create an Express app that retrieves known Jira problems by using Jira's Jira REST API. Additionally, you connected to a React frontend to your Express application, to display the issues in the browser.
This app offers a simple illustration of what you can do using the Jira REST API. The application can be improved by adding features which allows you to keep notes of tasks that have been accomplished, perform sophisticated filtering and more.
Jeremy Holcombe
Editorial and Content Editor WordPress Web Developer and Content Writer. In addition to everything related to WordPress I love beach, golf and beaches along with watching films. Also, I have height problems ;).
The post first appeared on here
Article was posted on here